JavaScript: Return the minimum-maximum value of an array, after applying the provided function to set comparing rule
JavaScript fundamental (ES6 Syntax): Exercise-15 with Solution
Min-Max Value of Array with Function
Write a JavaScript program to return the minimum-maximum value of an array, after applying the provided function to set a comparing rule.
- Use Array.prototype.reduce() in combination with the comparator function to get the appropriate element in the array.
- Omit the second argument, comparator, to use the default one that returns the minimum element in the array.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `reduce_Which` that returns the minimum element of an array based on a provided comparator function.
const reduce_Which = (arr, comparator = (a, b) => a - b) =>
arr.reduce((a, b) => (comparator(a, b) >= 0 ? b : a)); // Reduce the array and return the element that satisfies the comparator
// Example usage
console.log(reduce_Which([1, 3, 2])); // Returns the minimum element of the array
console.log(reduce_Which([10, 30, 20], (a, b) => b - a)); // Returns the maximum element of the array
console.log(reduce_Which(
[{ name: 'Kevin', age: 16 }, { name: 'John', age: 20 }, { name: 'Ani', age: 19 }],
(a, b) => a.age - b.age)); // Returns the object with the minimum age
Output:
1 30 {"name":"Kevin","age":16}
Flowchart:
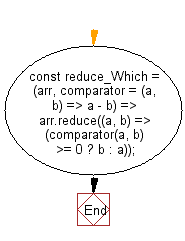
Live Demo:
See the Pen javascript-basic-exercise-1-15 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that applies a given function to each element of an array and returns both the minimum and maximum results.
- Write a JavaScript function that maps an array using a comparator function, then extracts the min and max values.
- Write a JavaScript program that finds the minimum and maximum of an array after transforming its elements via a provided callback.
Go to:
PREV : Replace Multiple Object Keys.
NEXT : Predicate Function Check for All Elements.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.