JavaScript: Returns true if the provided predicate function returns true for all elements in a collection, false otherwise
JavaScript fundamental (ES6 Syntax): Exercise-16 with Solution
Predicate Function Check for All Elements
Write a JavaScript function that returns true if the provided predicate function returns true for all elements in a collection, false otherwise.
- Use Array.prototype.every() to test if all elements in the collection return true based on fn.
- Omit the second argument, fn, to use Boolean as a default.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `all` that checks if all elements of an array satisfy a given condition.
const all = (arr, fn = Boolean) => arr.every(fn); // Check if every element in the array satisfies the provided condition
// Example usage
console.log(all([4, 2, 3], x => x > 1)); // Returns true since all elements are greater than 1
console.log(all([4, 2, 3], x => x < 1)); // Returns false since not all elements are less than 1
console.log(all([1, 2, 3])); // Returns true since all elements are truthy
Output:
true false true
Flowchart:
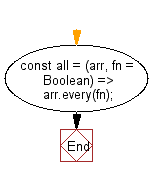
Live Demo:
See the Pen javascript-basic-exercise-1-16 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if a predicate function returns true for every element in an array.
- Write a JavaScript function that iterates over a collection and validates that all items satisfy a given condition.
- Write a JavaScript program that uses the Array.every() method to check if all elements meet the criteria specified by a predicate function.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to return the minimum-maximum value of an array, after applying the provided function to set comparing rule.
Next: Write a JavaScript program to split values of two given arrays into two groups. If an element in filter is truthy, the corresponding element in the collection belongs to the first group; otherwise, it belongs to the second group.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.