JavaScript: Split values of two given arrays into two groups
JavaScript fundamental (ES6 Syntax): Exercise-17 with Solution
Split Array into Two Groups
Write a JavaScript program to split the values of two given arrays into two groups. If an element in the filter is true, the corresponding element in the collection belongs to the first group; otherwise, it belongs to the second group.
- Use Array.prototype.reduce() and Array.prototype.push() to add elements to groups, based on filter.
- If filter has a truthy value for any element, add it to the first group, otherwise add it to the second group.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `bifurcate` that splits an array into two groups based on a filter array.
const bifurcate = (arr, filter) =>
arr.reduce((acc, val, i) => (acc[filter[i] ? 0 : 1].push(val), acc), [[], []]); // Split the array based on the filter array
// Example usage
console.log(bifurcate([1, 2, 3, 4], [true, true, false, true])); // [[1, 2, 4], [3]]
console.log(bifurcate([1, 2, 3, 4], [true, true, true, true])); // [[1, 2, 3, 4], []]
console.log(bifurcate([1, 2, 3, 4], [false, false, false, false])); // [[], [1, 2, 3, 4]]
Output:
[[1,2,4],[3]] [[1,2,3,4],[]] [[],[1,2,3,4]]
Flowchart:
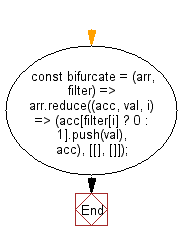
Live Demo:
See the Pen javascript-basic-exercise-1-17 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that splits an array into two groups based on a boolean filter array.
- Write a JavaScript function that separates elements into two arrays depending on whether a provided predicate function returns true or false.
- Write a JavaScript program that groups an array into two arrays based on a condition specified by a corresponding filter array.
Go to:
PREV : Predicate Function Check for All Elements.
NEXT : Remove Left Elements from Array.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.