JavaScript: Takes a predicate and array, like Array.filter(), but only keeps x if pred(x) returns false
JavaScript: Exercise-155 with Solution
Filter Where Predicate is False
Write a JavaScript program that takes a predicate and an array, like Array.filter(), but only keeps x if pred(x) returns false.
Filters an array's values based on a predicate function, returning only values for which the predicate function returns false.
- Use Array.prototype.filter() in combination with the predicate function, pred, to return only the values for which it returns false.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'reject' that filters elements from an array based on a provided predicate function
const reject = (pred, array) =>
// Filter the array using the negation of the predicate function
array.filter((...args) => !pred(...args));
// Test the 'reject' function with a predicate function and an array
console.log(reject(x => x % 2 === 0, [1, 2, 3, 4, 5])); // Output: [1, 3, 5]
console.log(reject(word => word.length > 4, ['Apple', 'Pear', 'Kiwi', 'Banana'])); // Output: ['Pear', 'Kiwi']
Output:
[1,3,5] ["Pear","Kiwi"]
Visual Presentation:
Flowchart:
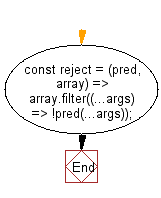
Live Demo:
See the Pen javascript-basic-exercise-155-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that replicates Array.filter() but only includes elements for which the predicate returns false.
- Write a JavaScript function that inverts a filtering predicate and returns an array of elements failing the test.
- Write a JavaScript program that processes an array to return only those items that do not satisfy a provided condition.
- Write a JavaScript function that filters an array for elements where a given function returns false and then logs the result.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create an object composed of the properties the given function returns falsey for. The function is invoked with two arguments: (value, key).
Next: Write a JavaScript program to apply a function against an accumulator and each element in the array (from left to right), returning an array of successively reduced values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.