JavaScript: Get a boolean determining if the passed value is primitive or not
JavaScript fundamental (ES6 Syntax): Exercise-190 with Solution
Check If Primitive
Write a JavaScript program to return a boolean determining if the passed value is primitive or not.
- Create an object from val and compare it with val to determine if the passed value is primitive (i.e. not equal to the created object).
Sample Solution:
JavaScript Code:
// Define a function 'isPrimitive' that checks if the given value 'val' is a primitive type
const isPrimitive = val =>
// Check if the type of the value is not 'object' or 'function', or if the value is null
!['object', 'function'].includes(typeof val) || val === null;
// Test cases to check if the values are primitive types
console.log(isPrimitive(null)); // true (null is a primitive type)
console.log(isPrimitive(50)); // true (number is a primitive type)
console.log(isPrimitive('Hello!')); // true (string is a primitive type)
console.log(isPrimitive(false)); // true (boolean is a primitive type)
console.log(isPrimitive(Symbol())); // true (symbol is a primitive type)
console.log(isPrimitive([])); // false (array is not a primitive type)
Output:
true true true true true false
Flowchart:
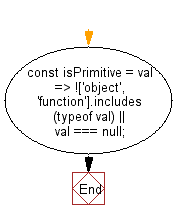
Live Demo:
See the Pen javascript-basic-exercise-190-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if a given value is a primitive type (string, number, boolean, symbol, null, or undefined).
- Write a JavaScript function that checks if an input is not an object or function and returns a boolean accordingly.
- Write a JavaScript program that distinguishes between primitive and reference types using typeof and instanceof.
- Write a JavaScript function that verifies if a variable is one of the known primitive types, excluding objects.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will return true if an object looks like a Promise, false otherwise.
Next: Write a JavaScript program to check if the provided integer is a prime number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.