JavaScript: Check whether the provided integer is a prime number or not
JavaScript fundamental (ES6 Syntax): Exercise-191 with Solution
Write a JavaScript program to check if the provided integer is a prime number or is not.
- Check numbers from 2 to the square root of the given number.
- Return false if any of them divides the given number, else return true, unless the number is less than 2.
Sample Solution:
JavaScript Code:
// Define a function 'isPrime' that checks if the given number 'num' is a prime number
const isPrime = num => {
// Define the boundary as the square root of the number rounded down to the nearest integer
const boundary = Math.floor(Math.sqrt(num));
// Iterate through numbers from 2 to the square root of 'num'
for (var i = 2; i <= boundary; i++)
// If 'num' is divisible by 'i' with no remainder, it's not a prime number, return false
if (num % i === 0) return false;
// Return true if 'num' is greater than or equal to 2, otherwise return false
return num >= 2;
};
// Test cases to check if the numbers are prime
console.log(isPrime(11)); // true (11 is a prime number)
console.log(isPrime(17)); // true (17 is a prime number)
console.log(isPrime(8)); // false (8 is not a prime number)
Output:
true true false
Visual Presentation:
Flowchart:
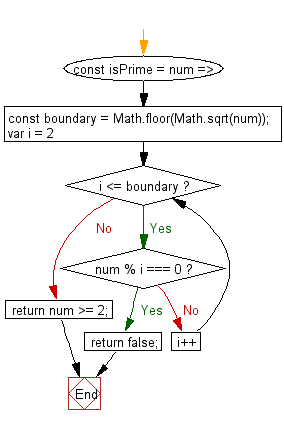
Live Demo:
See the Pen javascript-basic-exercise-191-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get a boolean determining if the passed value is primitive or not.
Next: Write a JavaScript program to check whether the provided value is an object created by the Object constructor.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-191.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics