JavaScript: Get a list of elements that exist in both arrays, after applying the provided function to each array element of both
JavaScript fundamental (ES6 Syntax): Exercise-210 with Solution
Common Elements After Mapping
Write a JavaScript program to get a list of elements in both arrays, after applying the provided function to each array element of both.
- Use Array.prototype.filter() and Array.prototype.findIndex() in combination with the provided comparator to determine intersecting values
Sample Solution:
JavaScript Code:
// Define a function 'intersectionBy' that computes the intersection of two arrays based on a transformation function
const intersectionBy = (a, b, fn) => {
// Create a Set 's' containing the transformed elements of array 'b' using the provided function 'fn'
const s = new Set(b.map(x => fn(x)));
// Filter elements from array 'a' if their transformed values exist in the Set 's'
return a.filter(x => s.has(fn(x)));
};
// Test the 'intersectionBy' function by finding the intersection of two arrays using the 'Math.floor' function as the transformation function
console.log(intersectionBy([2.1, 1.2], [2.3, 3.4], Math.floor));
// Output: [2.1]
Output:
[2.1]
Flowchart:
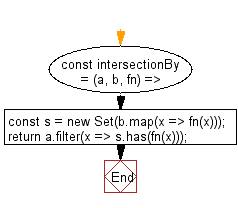
Live Demo:
See the Pen javascript-basic-exercise-210-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that maps each element of two arrays using a provided function, then returns the common elements.
- Write a JavaScript function that applies a transformation to two arrays and finds their intersection.
- Write a JavaScript program that uses a mapping function to normalize array elements before computing common values.
- Write a JavaScript function that returns an array of elements that match after applying a custom mapping to both input arrays.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get a list of elements that exist in both arrays, using a provided comparator function.
Next: Write a JavaScript program to get a list of elements that exist in both arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.