JavaScript: Filter out the non-unique values in an array
JavaScript fundamental (ES6 Syntax): Exercise-22 with Solution
Filter Non-Unique Values in Array
Write a JavaScript program to filter out non-unique values in an array.
- Use new Set() and the spread operator (...) to create an array of the unique values in arr.
- Use Array.prototype.filter() to create an array containing only the unique values.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `filter_Non_Unique` that filters an array and returns only the elements that are not unique.
const filter_Non_Unique = arr => arr.filter(i => arr.indexOf(i) === arr.lastIndexOf(i));
// Example usage
console.log(filter_Non_Unique([1, 2, 2, 3, 4, 4, 5]));
console.log(filter_Non_Unique([1, 2, 3, 4]));
Output:
[1,3,5] [1,2,3,4]
Visual Presentation:
Flowchart:
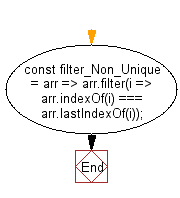
Live Demo:
See the Pen javascript-basic-exercise-1-22 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that filters out duplicate values from an array, returning only the unique ones.
- Write a JavaScript function that uses a frequency counter to eliminate non-unique elements from an array.
- Write a JavaScript program that returns an array containing only elements that occur exactly once in the original array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get every nth element in an given array.
Next: Write a JavaScript program to filter out the non-unique values in an array, based on a provided comparator function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.