JavaScript: Get the standard deviation of an array of numbers
JavaScript fundamental (ES6 Syntax): Exercise-225 with Solution
Standard Deviation
Write a JavaScript program to get the standard deviation of an array of numbers.
- Use Array.prototype.reduce() to calculate the mean, variance and the sum of the variance of the values and determine the standard deviation.
- Omit the second argument, usePopulation, to get the sample standard deviation or set it to true to get the population standard deviation.
Sample Solution:
JavaScript Code:
// Define a function 'standardDeviation' to calculate the standard deviation of an array
const standardDeviation = (arr, usePopulation = false) => {
// Calculate the mean of the array
const mean = arr.reduce((acc, val) => acc + val, 0) / arr.length;
// Calculate the sum of squared differences from the mean
const sumOfSquaredDifferences = arr.reduce((acc, val) => acc.concat((val - mean) ** 2), [])
.reduce((acc, val) => acc + val, 0);
// Calculate the standard deviation
return Math.sqrt(sumOfSquaredDifferences / (arr.length - (usePopulation ? 0 : 1)));
};
// Log the standard deviation of the array with and without using population adjustment
console.log(standardDeviation([10, 2, 38, 23, 38, 23, 21]));
console.log(standardDeviation([10, 2, 38, 23, 38, 23, 21], true));
Output:
13.284434142114991 12.29899614287479
Flowchart:
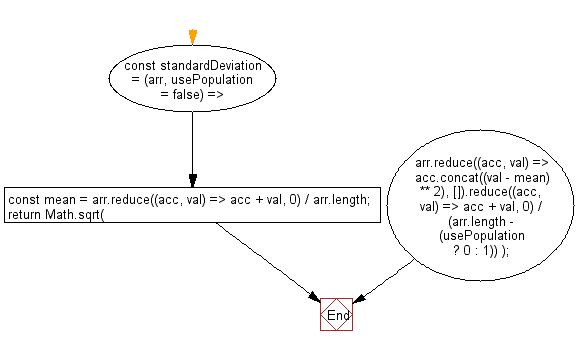
Live Demo:
See the Pen javascript-basic-exercise-225-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that computes the standard deviation of an array of numbers using statistical formulas.
- Write a JavaScript function that calculates the variance and then the standard deviation of a dataset.
- Write a JavaScript program that iterates over an array to compute the mean and standard deviation with a single pass algorithm.
- Write a JavaScript function that returns both the standard deviation and variance of an input array.
Go to:
PREV : Remove HTML/XML Tags.
NEXT : Remove Elements by Function.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.