JavaScript: Get the human readable format of the given number of milliseconds
JavaScript fundamental (ES6 Syntax): Exercise-230 with Solution
Milliseconds to Human Format
Write a JavaScript program to generate the human-readable format in the given number of milliseconds.
- Divide ms with the appropriate values to obtain the appropriate values for day, hour, minute, second and millisecond.
- Use Object.entries() with Array.prototype.filter() to keep only non-zero values.
- Use Array.prototype.map() to create the string for each value, pluralizing appropriately.
- Use String.prototype.join(', ') to combine the values into a string.
Sample Solution:
JavaScript Code:
// Define a function 'formatDuration' that converts milliseconds to a human-readable duration format
const formatDuration = ms => {
// If milliseconds are negative, convert them to positive
if (ms < 0) ms = -ms;
// Define an object 'time' to store the duration components
const time = {
day: Math.floor(ms / 86400000), // Calculate number of days
hour: Math.floor(ms / 3600000) % 24, // Calculate number of hours (mod 24 to get the remaining hours)
minute: Math.floor(ms / 60000) % 60, // Calculate number of minutes (mod 60 to get the remaining minutes)
second: Math.floor(ms / 1000) % 60, // Calculate number of seconds (mod 60 to get the remaining seconds)
millisecond: Math.floor(ms) % 1000 // Calculate number of milliseconds (mod 1000 to get the remaining milliseconds)
};
// Convert the 'time' object to an array of key-value pairs, filter out components with a value of 0, and format each component
return Object.entries(time)
.filter(val => val[1] !== 0) // Filter out components with a value of 0
.map(val => val[1] + ' ' + (val[1] !== 1 ? val[0] + 's' : val[0])) // Format each component
.join(', '); // Join the formatted components with a comma and space
};
// Test cases
console.log(formatDuration(1001)); // Output: "1 second, 1 millisecond"
console.log(formatDuration(34325055574)); // Output: "397 days, 6 hours, 9 minutes, 15 seconds, 574 milliseconds"
Output:
1 second, 1 millisecond 397 days, 6 hours, 44 minutes, 15 seconds, 574 milliseconds
Flowchart:
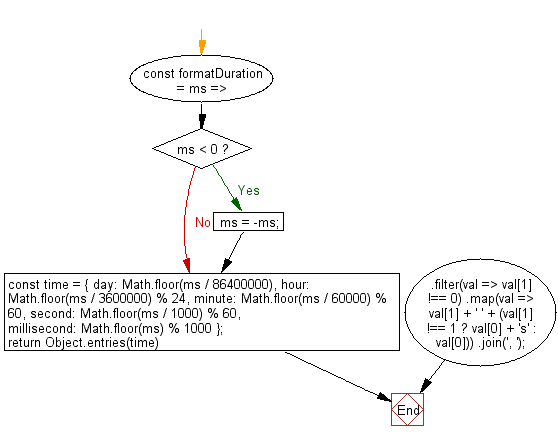
Live Demo:
See the Pen javascript-basic-exercise-230-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a number of milliseconds into a human-readable string in the format "X days, Y hours, Z minutes, W seconds".
- Write a JavaScript function that breaks down milliseconds into days, hours, minutes, and seconds with proper pluralization.
- Write a JavaScript program that formats a millisecond value into a concise human-readable duration.
- Write a JavaScript function that converts milliseconds to a string displaying time in a "HH:MM:SS" format, adjusting for days if necessary.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to convert a string from camelcase.
Next: Write a JavaScript program to iterate over all own properties of an object in reverse, running a callback for each one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.