JavaScript: Generate an array, containing the Fibonacci sequence, up until the nth term
JavaScript fundamental (ES6 Syntax): Exercise-237 with Solution
Fibonacci Sequence
Write a JavaScript program to generate an array containing the Fibonacci sequence, up to the nth term.
- Use Array.from() to create an empty array of the specific length, initializing the first two values (0 and 1).
- Use Array.prototype.reduce() and Array.prototype.concat() to add values into the array, using the sum of the last two values, except for the first two.
Sample Solution:
JavaScript Code:
// Define a function 'fibonacci' to generate Fibonacci sequence up to the specified length
const fibonacci = n =>
Array.from({ length: n }).reduce((acc, val, i) =>
acc.concat(i > 1 ? acc[i - 1] + acc[i - 2] : i), []); // Generate Fibonacci sequence
// Test the 'fibonacci' function with different lengths
console.log(fibonacci(2)); // Output: [0, 1]
console.log(fibonacci(3)); // Output: [0, 1, 1]
console.log(fibonacci(6)); // Output: [0, 1, 1, 2, 3, 5]
Output:
[0,1] [0,1,1] [0,1,1,2,3,5]
Flowchart:
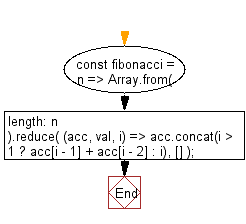
Live Demo:
See the Pen javascript-basic-exercise-237-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that generates an array containing the Fibonacci sequence up to the nth term recursively.
- Write a JavaScript function that builds the Fibonacci sequence iteratively and returns an array of numbers.
- Write a JavaScript program that uses memoization to efficiently generate the Fibonacci sequence for large n.
- Write a JavaScript function that outputs the Fibonacci sequence in a functional programming style using reduce().
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the first key that satisfies the provided testing function. Otherwise return undefined.
Next: Write a JavaScript program to calculate the factorial of a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.