JavaScript: Calculate the factorial of a number
JavaScript fundamental (ES6 Syntax): Exercise-238 with Solution
Factorial of Number
Write a JavaScript program to calculate the factorial of a number.
- Use recursion.
- If n is less than or equal to 1, return 1.
- Otherwise, return the product of n and the factorial of n - 1.
- Throw a TypeError if n is a negative number.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'factorial' to calculate the factorial of a given number 'n'
const factorial = n =>
// If 'n' is less than 0, throw a TypeError indicating that negative numbers are not allowed
n < 0
? (() => {
throw new TypeError('Negative numbers are not allowed!');
})()
// If 'n' is 0 or 1, return 1 (base case of factorial)
: n <= 1
? 1
// Otherwise, recursively calculate the factorial of 'n' using the formula n * factorial(n - 1)
: n * factorial(n - 1);
// Test the 'factorial' function with different inputs
console.log(factorial(1)); // Output: 1
console.log(factorial(5)); // Output: 120 (1 * 2 * 3 * 4 * 5)
console.log(factorial(7)); // Output: 5040 (1 * 2 * 3 * 4 * 5 * 6 * 7)
Output:
1 120 5040
Visual Presentation:
Flowchart:
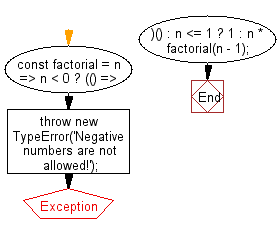
Live Demo:
See the Pen javascript-basic-exercise-238-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that calculates the factorial of a number recursively.
- Write a JavaScript function that computes a factorial iteratively using a for loop.
- Write a JavaScript program that uses reduce() to calculate the factorial of a given integer.
- Write a JavaScript function that implements tail recursion to compute the factorial of a large number.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to generate an array, containing the Fibonacci sequence, up until the nth term.
Next: Write a JavaScript program to escape a string to use in a regular expression.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.