JavaScript: Remove elements in an array until the passed function returns true
JavaScript fundamental (ES6 Syntax): Exercise-241 with Solution
Remove Elements Until Condition
Write a JavaScript program to remove elements from an array until the passed function returns true. Returns the remaining elements in the array.
- Loop through the array, using Array.prototype.slice() to drop the first element of the array until the value returned from func is true.
- Return the remaining elements.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'dropWhile' to remove elements from the beginning of an array until a condition is met
const dropWhile = (arr, func) => {
// Iterate over the array while its length is greater than 0 and the condition for the first element fails
while (arr.length > 0 && !func(arr[0]))
// Remove the first element from the array
arr = arr.slice(1);
// Return the modified array
return arr;
};
// Test the 'dropWhile' function with an array and a condition function
console.log(dropWhile([1, 2, 3, 4], n => n >= 3)); // Output: [3, 4]
Output:
[3,4]
Flowchart:
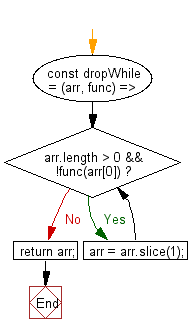
Live Demo:
See the Pen javascript-basic-exercise-241-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that removes elements from the start of an array until a provided condition is met and returns the remaining array.
- Write a JavaScript function that uses a while loop to shift elements off an array until a predicate returns true.
- Write a JavaScript program that processes an array and stops removing items once an element satisfies a test.
- Write a JavaScript function that returns the array after all initial elements failing a condition are removed.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will return true if the parent element contains the child element, false otherwise.
Next: Write a JavaScript program to remove elements from the end of an array until the passed function returns true. Returns the remaining elements in the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.