JavaScript: Return true if the string is y/yes other wise false
JavaScript fundamental (ES6 Syntax): Exercise-26 with Solution
Check y/yes or n/no in String
Write a JavaScript program that returns true if the string is y/yes or false if the string is n/no.
- Use RegExp.prototype.test() to check if the string evaluates to y/yes or n/no.
- Omit the second argument, def to set the default answer as no.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `yes_No` that checks if the input string represents a "yes" or "no" value.
// It returns `true` for "yes", `false` for "no", and the default value if neither "yes" nor "no" is found.
const yes_No = (val, def = false) =>
/^(y|yes)$/i.test(val) ? true : /^(n|no)$/i.test(val) ? false : def;
// Example usage
console.log(yes_No('Y')); // true
console.log(yes_No('yes')); // true
console.log(yes_No('No')); // false
console.log(yes_No('Foo', true)); // true (default value)
Note: Omit the second argument, def to set the default answer as no.
Output:
true true false true
Visual Presentation:
Flowchart:
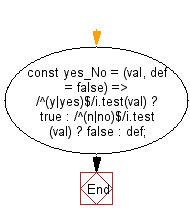
Live Demo:
See the Pen javascript-basic-exercise-1-26 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if a string equals "y" or "yes" (case-insensitive) and false for "n" or "no".
- Write a JavaScript function that normalizes input strings and checks for affirmative or negative responses.
- Write a JavaScript program that trims and lowercases a string before determining if it matches "y/yes" or "n/no".
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create a new array out of the two supplied by creating each possible pair from the arrays.
Next: Write a JavaScript program to find every element that exists in any of the two given arrays once, using a provided comparator function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.