JavaScript: Find all elements in a given array except for the first one
JavaScript fundamental (ES6 Syntax): Exercise-31 with Solution
All Elements Except First in Array
Write a JavaScript program to find all elements in a given array except the first one. Return the whole array if its length is 1.
- Return Array.prototype.slice(1) if Array.prototype.length is more than 1, otherwise, return the whole array.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function named tail that returns all elements of an array except the first one.
const tail = arr => (arr.length > 1 ? arr.slice(1) : arr);
// Test the function with different input arrays.
console.log(tail([1, 2, 3])); // Output: [2, 3]
console.log(tail([1])); // Output: [1] (unchanged because the array has only one element)
Output:
[2,3] [1]
Visual Presentation:
Flowchart:
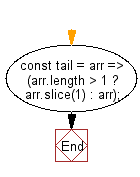
Live Demo:
See the Pen javascript-basic-exercise-1-31 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to filter out the element(s) of a given array, that have one of the specified values.
Next: Write a JavaScript program to get the sum of a given array, after mapping each element to a value using the provided function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-31.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics