JavaScript: Create a function that invokes each provided function with the arguments it receives and returns the results
JavaScript fundamental (ES6 Syntax): Exercise-36 with Solution
Invoke Functions with Arguments
Write a JavaScript program to create a function that invokes each provided function with the arguments it receives and returns the results.
- Use Array.prototype.map() and Function.prototype.apply() to apply each function to the given arguments.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a higher-order function named 'over' that takes multiple functions as arguments and returns a new function.
const over = (...fns) => (...args) => fns.map(fn => fn.apply(null, args));
// Create a new function named 'minMax' using the 'over' function to combine 'Math.min' and 'Math.max'.
const minMax = over(Math.min, Math.max);
// Test the 'minMax' function with different sets of numbers and output the results.
console.log(minMax(1, 2, 3, 4, 5)); // Output: [1, 5] (minimum and maximum values)
console.log(minMax(1, 2, 5, 4, 3)); // Output: [1, 5] (minimum and maximum values)
console.log(minMax(1, 2, 5, -4, 3)); // Output: [-4, 5] (minimum and maximum values)
Output:
[1,5] [1,5] [-4,5]
Visual Presentation:
Flowchart:
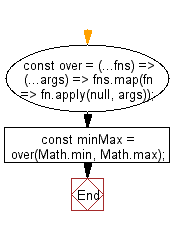
Live Demo:
See the Pen javascript-basic-exercise-1-36 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that takes an array of functions and invokes each with the provided arguments, returning an array of results.
- Write a JavaScript function that iterates over multiple callback functions and applies the same set of arguments to each.
- Write a JavaScript program that uses the spread operator to pass arguments to a list of functions and collect their outputs.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get an array of given n random integers in the specified range.
Next: Write a JavaScript program to get a sorted array of objects ordered by properties and orders.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.