JavaScript fundamental: Get a sorted array of objects ordered by properties and orders
JavaScript fundamental (ES6 Syntax): Exercise-37 with Solution
Sort Array of Objects by Properties
Write a JavaScript program to get a sorted array of objects ordered by properties and orders.
- Uses Array.prototype.sort(), Array.prototype.reduce() on the props array with a default value of 0.
- Use array destructuring to swap the properties position depending on the order supplied.
- If no orders array is supplied, sort by 'asc' by default.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'orderBy' that takes three parameters: 'arr' (an array), 'props' (an array of property names), and 'orders' (an optional array of sort orders).
const orderBy = (arr, props, orders) =>
// Create a new array by cloning 'arr', then sort it based on the given properties and orders.
[...arr].sort((a, b) =>
// Reduce the properties to a single value that represents the comparison result.
props.reduce((acc, prop, i) => {
if (acc === 0) {
// Check if the current property should be sorted in descending order.
const [p1, p2] = orders && orders[i] === 'desc' ? [b[prop], a[prop]] : [a[prop], b[prop]];
// Compare the properties and update the accumulator accordingly.
acc = p1 > p2 ? 1 : p1 < p2 ? -1 : 0;
}
return acc; // Return the final comparison result.
}, 0) // Start with an initial value of 0 for the accumulator.
);
// Define an array of user objects.
const users = [
{ name: 'fred', age: 48 },
{ name: 'barney', age: 36 },
{ name: 'fred', age: 40 }
];
// Test the 'orderBy' function with different property names and sort orders, then output the sorted arrays.
console.log(orderBy(users, ['name', 'age'], ['asc', 'desc']));
console.log(orderBy(users, ['name', 'age']));
Output:
[{"name":"barney","age":36},{"name":"fred","age":48},{"name":"fred","age":40}] [{"name":"barney","age":36},{"name":"fred","age":40},{"name":"fred","age":48}]
Flowchart:
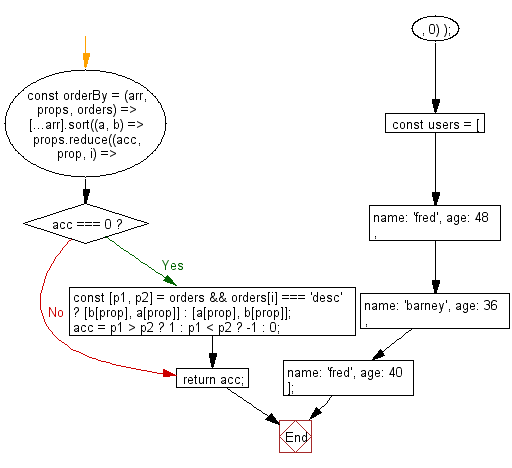
Live Demo:
See the Pen javascript-basic-exercise-1-37 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create a function that invokes each provided function with the arguments it receives and returns the results.
Next: Write a JavaScript program to pad a string on both sides with the specified character, if it's shorter than the specified length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-37.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics