JavaScript: Perform right-to-left function composition
JavaScript fundamental (ES6 Syntax): Exercise-66 with Solution
Right-to-Left Function Composition
Write a JavaScript program to perform right-to-left function composition.
- Use Array.prototype.reduce() to perform right-to-left function composition.
- The last (rightmost) function can accept one or more arguments; the remaining functions must be unary.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'compose' that takes any number of functions as arguments and returns a new function.
const compose = (...fns) => fns.reduce((f, g) => (...args) => f(g(...args)));
// Define functions 'add5' and 'multiply'.
const add5 = x => x + 5;
const multiply = (x, y) => x * y;
// Create a new function 'multiplyAndAdd5' by composing 'add5' and 'multiply'.
const multiplyAndAdd5 = compose(
add5,
multiply
);
// Example usage:
console.log(multiplyAndAdd5(5, 2)); // Output 15
Output:
15
Visual Presentation:
Flowchart:
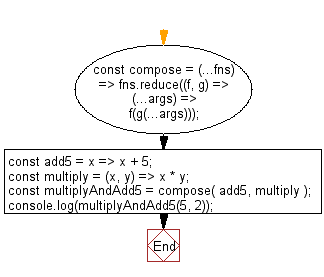
Live Demo:
See the Pen javascript-basic-exercise-66-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that composes multiple functions from right to left and returns the composed function.
- Write a JavaScript function that accepts a list of functions and creates a new function that applies them in reverse order.
- Write a JavaScript program that demonstrates right-to-left function composition using array.reduceRight().
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to add special characters to text to print in color in the console (combined with console.log()).
Next: Write a JavaScript program to perform left-to-right function composition.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.