JavaScript: Accepts a converging function and a list of branching functions
JavaScript fundamental (ES6 Syntax): Exercise-68 with Solution
Converge Function with Branching Functions
Write a JavaScript program that accepts a converging function and a list of branching functions. It returns a function that applies each branching function to the arguments. The results of the branching functions are passed as arguments to the converging function.
- Use Array.prototype.map() and Function.prototype.apply() to apply each function to the given arguments.
- Use the spread operator (...) to call converger with the results of all other functions.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'converge' that takes a 'converger' function and an array of functions 'fns' as arguments and returns a new function.
const converge = (converger, fns) => (...args) =>
converger(...fns.map(fn => fn.apply(null, args)));
// Define functions 'average' and 'sum'.
const average = converge((a, b) => a / b, [
arr => arr.reduce((a, v) => a + v, 0),
arr => arr.length
]);
// Example usage:
console.log(average([6, 7])); // Output: 6.5
console.log(average([1, 2, 3, 4, 5, 6, 7])); // Output: 4
Output:
6.5 4
Visual Presentation:
Flowchart:
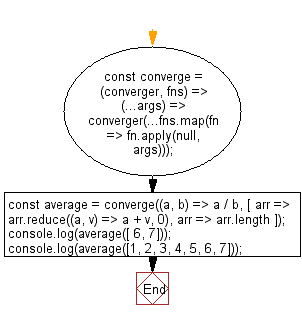
Live Demo:
See the Pen javascript-basic-exercise-68-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that accepts multiple branching functions and a converging function to merge their outputs.
- Write a JavaScript function that applies several functions to the same arguments and then passes all results to a final function.
- Write a JavaScript program that demonstrates convergent composition by processing inputs through parallel functions and combining their outputs.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to perform left-to-right function composition.
Next: Write a JavaScript program to group the elements of an array based on the given function and returns the count of elements in each group.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.