JavaScript: Create a deep clone of an object
JavaScript fundamental (ES6 Syntax): Exercise-71 with Solution
Deep Clone Object
Write a JavaScript program to create a deep clone of an object.
- Use recursion.
- Check if the passed object is null and, if so, return null.
- Use Object.assign() and an empty object ({}) to create a shallow clone of the original.
- Use Object.keys() and Array.prototype.forEach() to determine which key-value pairs need to be deep cloned.
- If the object is an Array, set the clone's length to that of the original and use Array.from(clone) to create a clone.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'deepClone' to create a deep clone of an object.
const deepClone = obj => {
// Create a shallow clone of the object using Object.assign
let clone = Object.assign({}, obj);
// Iterate over the keys of the clone
Object.keys(clone).forEach(
key => {
// If the value of the key is an object, recursively call deepClone
clone[key] = typeof obj[key] === 'object' ? deepClone(obj[key]) : obj[key];
}
);
// If the original object is an array, update the length of the clone and convert it to an array
return Array.isArray(obj) ? (clone.length = obj.length) && Array.from(clone) : clone;
};
// Example usage:
const a = { foo: 'bar', obj: { a: 1, b: 2 } };
const b = deepClone(a);
console.log(b);
Output:
{"foo":"bar","obj":{"a":1,"b":2}}
Flowchart:
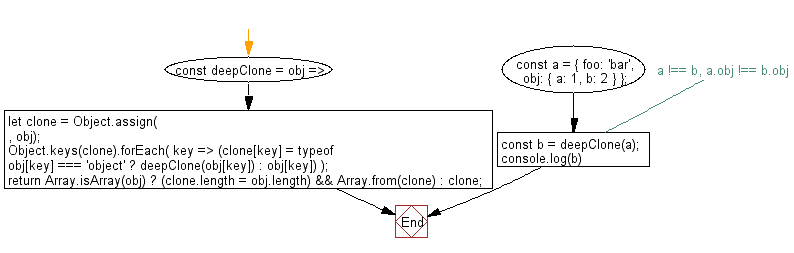
Live Demo:
See the Pen javascript-basic-exercise-71-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates a deep clone of an object, including nested objects and arrays.
- Write a JavaScript function that recursively copies all properties of an object to produce an independent clone.
- Write a JavaScript program that compares a deep-cloned object with the original to ensure no references are shared.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to count the occurrences of a value in an array.
Next: Write a JavaScript program to detect if the website is being opened in a mobile device or a desktop/laptop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.