JavaScript: Return the difference between two arrays
JavaScript fundamental (ES6 Syntax): Exercise-73 with Solution
Difference Between Two Arrays with Mapping
Write a JavaScript program to return the difference between two arrays, after applying the provided function to each array element of both.
- Create a Set by applying fn to each element in b.
- Use Array.prototype.map() to apply fn to each element in a.
- Use Array.prototype.filter() in combination with fn on a to only keep values not contained in b, using Set.prototype.has().
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'differenceBy' to find the difference between two arrays based on a provided function.
const differenceBy = (a, b, fn) => {
// Create a Set containing the result of applying the function 'fn' to each element of array 'b'.
const s = new Set(b.map(v => fn(v)));
// Filter array 'a' to keep only those elements for which the result of applying 'fn' is not present in Set 's'.
return a.filter(x => !s.has(fn(x)));
};
// Example usage:
console.log(differenceBy([2.1, 1.2], [2.3, 3.4], Math.floor)); // Outputs: [1.2]
console.log(differenceBy([{ x: 2 }, { x: 1 }], [{ x: 1 }], v => v.x)); // Outputs: [{ x: 2 }]
Output:
[1.2] [{"x":2}]
Flowchart:
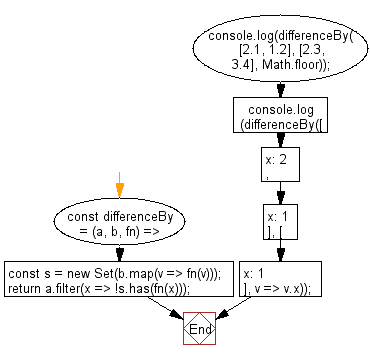
Live Demo:
See the Pen javascript-fundamental-exercise-73 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns the difference between two arrays after applying a mapping function to each element.
- Write a JavaScript function that computes the symmetric difference of two arrays based on transformed values.
- Write a JavaScript program that maps two arrays with a provided function and then returns elements that do not appear in both.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to detect if the website is being opened in a mobile device or a desktop/laptop.
Next: Write a JavaScript program to filter out all values from an array for which the comparator function does not return true.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.