JavaScript: Filter out all values from an array for which the comparator function does not return true
JavaScript fundamental (ES6 Syntax): Exercise-74 with Solution
Filter Array by Comparator
Write a JavaScript program to filter out all values from an array for which the comparator function does not return true.
- Use Array.prototype.filter() and Array.prototype.findIndex() to find the appropriate values.
- Omit the last argument, comp, to use a default strict equality comparator.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'differenceWith' to find the difference between two arrays based on a provided comparison function.
const differenceWith = (arr, val, comp) =>
// Filter array 'arr' to keep only those elements for which no element in array 'val' satisfies the comparison function 'comp'.
arr.filter(a => val.findIndex(b => comp(a, b)) === -1);
// Example usage:
console.log(differenceWith([1, 1.2, 1.5, 3, 0], [1.9, 3, 0], (a, b) => Math.round(a) === Math.round(b)));
Output:
[1,1.2]
Flowchart:
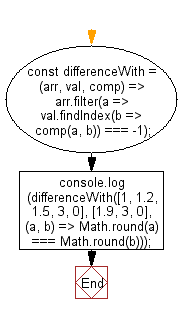
Live Demo:
See the Pen javascript-basic-exercise-74-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to return the difference between two arrays, after applying the provided function to each array element of both.
Next: Write a JavaScript program to compute the new ratings between two or more opponents using the Elo rating system.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-74.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics