JavaScript: Execute a provided function once for each array element, starting from the array's last element
JavaScript fundamental (ES6 Syntax): Exercise-76 with Solution
Execute Function for Array in Reverse Order
Write a JavaScript program to execute a provided function once for each array element, starting with the array's last element.
- Use Array.prototype.slice() to clone the given array and Array.prototype.reverse() to reverse it.
- Use Array.prototype.forEach() to iterate over the reversed array.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'forEachRight' to iterate over an array in reverse order and apply a callback function to each element.
const forEachRight = (arr, callback) =>
// Create a shallow copy of the array, reverse it, and iterate over each element.
arr
.slice(0) // Create a shallow copy of the array to avoid mutating the original array.
.reverse() // Reverse the order of elements in the copied array.
.forEach(callback); // Apply the callback function to each element.
// Example usage:
forEachRight([1, 2, 3, 4], val => console.log(val)); // Outputs: 4, 3, 2, 1
Output:
4 3 2 1
Flowchart:
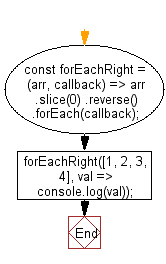
Live Demo:
See the Pen javascript-basic-exercise-76-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that iterates over an array from the last element to the first, applying a provided function.
- Write a JavaScript function that uses a reverse loop to execute a callback on each array element in reverse order.
- Write a JavaScript program that reverses an array and then invokes a function for each element, preserving the original array order.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to compute the new ratings between two or more opponents using the Elo rating system
Next: Write a JavaScript program to iterate over all own properties of an object, running a callback for each one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.