JavaScript: Get the median of an array of numbers
JavaScript fundamental (ES6 Syntax): Exercise-88 with Solution
Find Median of Numbers in Array
Write a JavaScript program to get the median of an array of numbers.
Note: Find the middle of the array, use Array.sort() to sort the values. Return the number at the midpoint if length is odd, otherwise the average of the two middle numbers.
- Find the middle of the array, use Array.prototype.sort() to sort the values.
- Return the number at the midpoint if Array.prototype.length is odd, otherwise the average of the two middle numbers.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the function 'median' to find the median value of an array.
const median = arr => {
const mid = Math.floor(arr.length / 2),
nums = [...arr].sort((a, b) => a - b);
return arr.length % 2 !== 0 ? nums[mid] : (nums[mid - 1] + nums[mid]) / 2;
};
// Example usage:
console.log(median([5, 6, 50, 1, -5])); // Outputs: 5 (median of the array)
console.log(median([1, 2, 3, 4, 5])); // Outputs: 3 (median of the array)
Output:
5 3
Visual Presentation:
Flowchart:
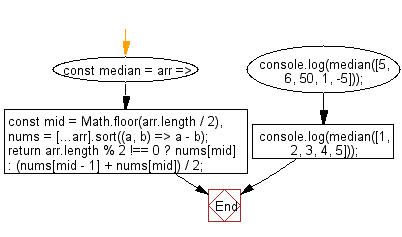
Live Demo:
See the Pen javascript-basic-exercise-88-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that calculates the median value of a numeric array after sorting it.
- Write a JavaScript function that handles both even and odd array lengths to return the median correctly.
- Write a JavaScript program that computes the median without fully sorting the array by using a selection algorithm.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the n maximum elements from the provided array. If n is greater than or equal to the provided array's length, then return the original array(sorted in descending order).
Next: Write a JavaScript program to negates a predicate function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.