JavaScript: Add an event listener to an element with the ability to use event delegation
JavaScript fundamental (ES6 Syntax): Exercise-95 with Solution
Add Event Listener with Delegation
Write a JavaScript program to add an event listener to an element with the ability to use event delegation.
- Use EventTarget.addEventListener() to add an event listener to an element.
- If there is a target property supplied to the options object, ensure the event target matches the target specified and then invoke the callback by supplying the correct this context.
- Omit opts to default to non-delegation behavior and event bubbling.
- Returns a reference to the custom delegator function, in order to be possible to use with off.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'on' function to attach an event listener to an element.
const on = (el, evt, fn, opts = {}) => {
// Define a delegator function if 'opts.target' is provided.
const delegatorFn = e => e.target.matches(opts.target) && fn.call(e.target, e);
// Add event listener to the element based on the options provided.
el.addEventListener(evt, opts.target ? delegatorFn : fn, opts.options || false);
// Return the delegator function if 'opts.target' is provided.
if (opts.target) return delegatorFn;
};
// Define a test function.
const fn = () => console.log('!');
// Attach a click event listener to the document body.
console.log(on(document.body, 'click', fn)); // Output: undefined
// Attach a click event listener to the document body, delegating it to <p> elements.
console.log(on(document.body, 'click', fn, { target: 'p' })); // Output: undefined
// Attach a click event listener to the document body with options.
console.log(on(document.body, 'click', fn, { options: true })); // Output: undefined
Output:
undefined undefined undefined
Flowchart:
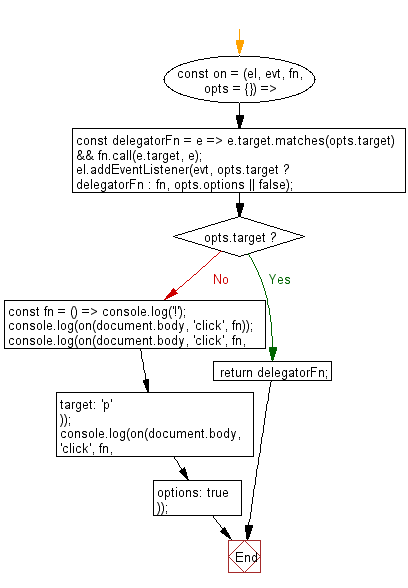
Live Demo:
See the Pen javascript-basic-exercise-95-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that adds an event listener to a parent element to handle events on its child elements using delegation.
- Write a JavaScript function that attaches a single click event listener to a container, processing events for dynamically added items.
- Write a JavaScript program that demonstrates event delegation by filtering event targets within a common ancestor element.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to move the specified amount of elements to the end of the array.
Next: Write a JavaScript program to pick the key-value pairs corresponding to the given keys from an object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.