JavaScript: Move the specified amount of elements to the end of the array
JavaScript fundamental (ES6 Syntax): Exercise-94 with Solution
Move Array Elements to End
Write a JavaScript program to move the specified amount of elements to the end of the array.
- Use Array.prototype.slice() twice to get the elements after the specified index and the elements before that.
- Use the spread operator (...) to combine the two into one array.
- If offset is negative, the elements will be moved from end to start.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'offset' function to offset the elements of an array by a specified amount.
const offset = (arr, offset) => [...arr.slice(offset), ...arr.slice(0, offset)];
// Offset the elements of the array by 2 positions to the right.
console.log(offset([1, 2, 3, 4, 5], 2)); // Output: [3, 4, 5, 1, 2]
// Offset the elements of the array by 2 positions to the left.
console.log(offset([1, 2, 3, 4, 5], -2)); // Output: [4, 5, 1, 2, 3]
Output:
[3,4,5,1,2] [4,5,1,2,3]
Visual Presentation:
Flowchart:
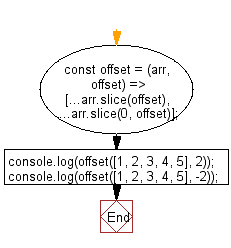
Live Demo:
See the Pen javascript-basic-exercise-94-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that moves a specified number of elements from the beginning of an array to its end.
- Write a JavaScript function that shifts the first n elements of an array and appends them at the end, preserving order.
- Write a JavaScript program that rotates an array left by n positions and returns the modified array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to remove an event listener from an element.
Next: Write a JavaScript program to add an event listener to an element with the ability to use event delegation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.