JavaScript: Randomly arrange or shuffle an array
JavaScript Array: Exercise-17 with Solution
Shuffle Array
Write a JavaScript program to shuffle an array.
Sample Solution:
JavaScript Code:
// Function to shuffle an array using the Fisher-Yates algorithm
function shuffle(arra1) {
// Initialize variables: ctr is the counter, temp is a temporary variable, index is the random index
var ctr = arra1.length, temp, index;
// While there are elements in the array
while (ctr > 0) {
// Pick a random index
index = Math.floor(Math.random() * ctr);
// Decrease ctr by 1
ctr--;
// Swap the last element with the randomly picked element
temp = arra1[ctr];
arra1[ctr] = arra1[index];
arra1[index] = temp;
}
// Return the shuffled array
return arra1;
}
// Original array for testing
var myArray = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9];
// Output the shuffled array
console.log(shuffle(myArray));
Output:
[5,3,0,9,8,2,1,4,7,6]
Flowchart:
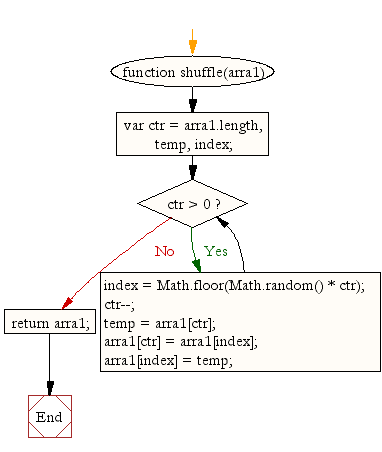
ES6 Version:
// Function to shuffle an array using the Fisher-Yates algorithm
const shuffle = (arra1) => {
// Initialize variables: ctr is the counter, temp is a temporary variable, index is the random index
let ctr = arra1.length, temp, index;
// While there are elements in the array
while (ctr > 0) {
// Pick a random index
index = Math.floor(Math.random() * ctr);
// Decrease ctr by 1
ctr--;
// Swap the last element with the randomly picked element
temp = arra1[ctr];
arra1[ctr] = arra1[index];
arra1[index] = temp;
}
// Return the shuffled array
return arra1;
};
// Original array for testing
const myArray = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9];
// Output the shuffled array
console.log(shuffle(myArray));
Live Demo:
See the Pen JavaScript - Randomly arrange or shuffle an array - array-ex- 17 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that shuffles an array using the Fisher-Yates algorithm for unbiased randomization.
- Write a JavaScript function that randomly rearranges an array and verifies randomness over multiple iterations.
- Write a JavaScript function that shuffles an array in place without creating a new array.
- Write a JavaScript function that ensures no element remains in its original index after shuffling.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to find the leap years from a given range of years
Next: Write a JavaScript program to perform a binary search.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.