JavaScript: Clone an array
JavaScript Array: Exercise-2 with Solution
Write a JavaScript function to clone an array.
Test Data:
console.log(array_Clone([1, 2, 4, 0]));
console.log(array_Clone([1, 2, [4, 0]]));
[1, 2, 4, 0]
[1, 2, [4, 0]]
Visual Presentation:
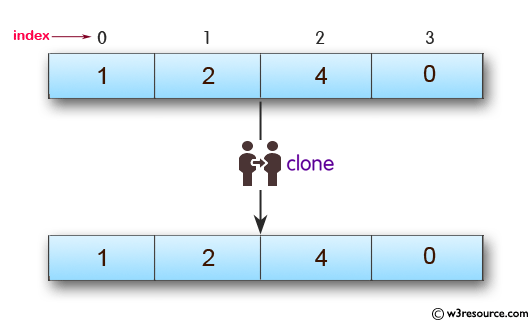
Sample Solution-1:
JavaScript Code:
// Function to clone an array
var array_Clone = function(arra1) {
// Using the slice method to create a shallow copy of the input array
return arra1.slice(0);
};
// Testing the function with an array
console.log(array_Clone([1, 2, 4, 0]));
// Testing the function with an array containing nested arrays
console.log(array_Clone([1, 2, [4, 0]]));
Output:
[1,2,4,0] [1,2,[4,0]]
Flowchart:
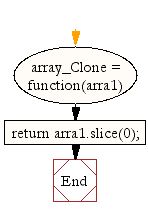
ES6 Version:
// Function to clone an array
const array_Clone = arra1 => {
// Using the slice method to create a shallow copy of the input array
return arra1.slice(0);
};
// Testing the function with an array
console.log(array_Clone([1, 2, 4, 0]));
// Testing the function with an array containing nested arrays
console.log(array_Clone([1, 2, [4, 0]]));
Sample Solution-2:
JavaScript provides quite a few ways to clone an array, most of which are pretty similar in terms of performance and results. Here's a quick rundown of some of the available options.
The spread operator:
ES6 introduced the spread operator (...), which provides probably the easiest and most common way to create a shallow clone of an array.
JavaScript Code:
let x = [1, 2, 3, 4];
console.log("Original array:")
console.log(x)
let y = [...x];
console.log("Clone of the said array:")
console.log(y)
Output:
Original array: [1,2,3,4] Clone of the said array: [1,2,3,4]
Sample Solution-3:
Array.from()
Array.from() has a very powerful API that can be used for many different things, including creating a copy of an array.
JavaScript Code:
let x = [1, 2, 3, 4];
console.log("Original array:")
console.log(x)
let y = Array.from(x);
console.log("Clone of the said array:")
console.log(y)
Output:
Original array: [1,2,3,4] Clone of the said array: [1,2,3,4]
Sample Solution-4:
Array.prototype.slice()
Similarly to the spread operator, Array.prototype.slice() can be used to create a shallow copy of an array.
JavaScript Code:
let x = [1, 2, 3, 4];
console.log("Original array:")
console.log(x)
let y = x.slice();
console.log("Clone of the said array:")
console.log(y)
Output:
Original array: [1,2,3,4] Clone of the said array: [1,2,3,4]
Sample Solution-5:
Array.prototype.map()
Looking into one of the more unorthodox options, Array.prototype.map() can be used to map each element of an array to itself to create a new array.
JavaScript Code:
let x = [1, 2, 3, 4];
console.log("Original array:")
console.log(x)
let y = x.map(i => i);
console.log("Clone of the said array:")
console.log(y)
Output:
Original array: [1,2,3,4] Clone of the said array: [1,2,3,4]
Sample Solution-6:
Array.prototype.filter()
Similarly, Array.prototype.filter() can be used to return true for each and every element, resulting in a new array with all of the original array's elements.
JavaScript Code:
let x = [1, 2, 3, 4];
console.log("Original array:")
console.log(x)
let y = x.filter(() => true);
console.log("Clone of the said array:")
console.log(y)
Output:
Original array: [1,2,3,4] Clone of the said array: [1,2,3,4]
Live Demo :
See the Pen JavaScript - Clone an array- array-ex-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to check whether an 'input' is an array or not.
Next: Write a JavaScript function to get the first element of an array. Passing a parameter 'n' will return the first 'n' elements of the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics