JavaScript: Get the first element of an array
JavaScript Array: Exercise-3 with Solution
First Elements of Array
Write a JavaScript function to get the first element of an array. Passing the parameter 'n' will return the first 'n' elements of the array.
Test Data:
console.log(array_Clone([1, 2, 4, 0]));
console.log(array_Clone([1, 2, [4, 0]]));
[1, 2, 4, 0]
[1, 2, [4, 0]]
Visual Presentation:
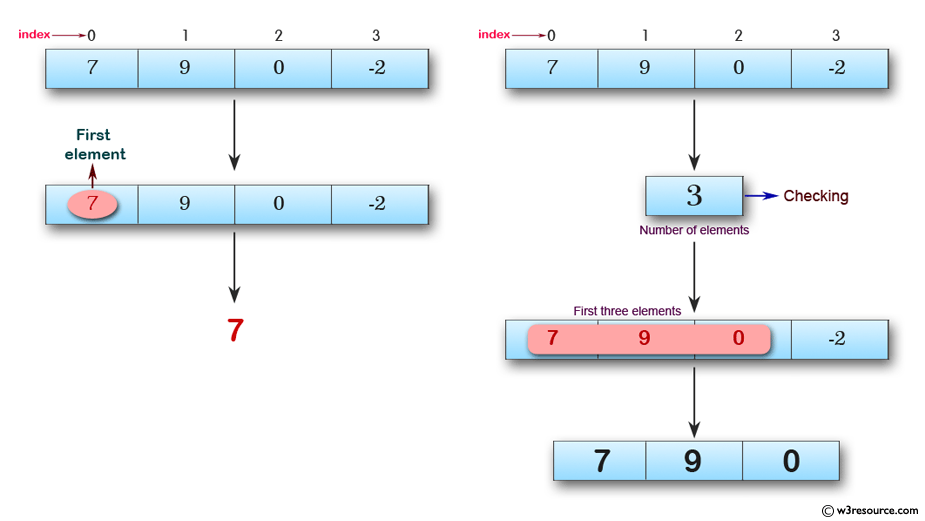
Sample Solution:
JavaScript Code:
// Function to get the first n elements of an array
var first = function(array, n) {
// Check if the input array is null, return undefined if true
if (array == null)
return void 0;
// Check if the value of n is null, return the first element of the array if true
if (n == null)
return array[0];
// Check if the value of n is negative, return an empty array if true
if (n < 0)
return [];
// Use the slice method to get the first n elements of the array
return array.slice(0, n);
};
// Testing the function with various cases
console.log(first([7, 9, 0, -2]));
console.log(first([], 3));
console.log(first([7, 9, 0, -2], 3));
console.log(first([7, 9, 0, -2], 6));
console.log(first([7, 9, 0, -2], -3));
Output:
7 [] [7,9,0] [7,9,0,-2] []
Flowchart:
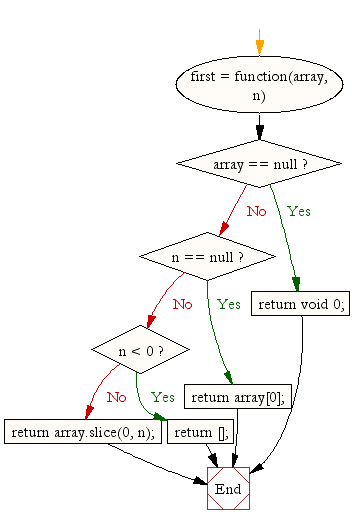
ES6 Version:
// Function to get the first n elements of an array
const first = (array, n) => {
// Check if the input array is null, return undefined if true
if (array == null)
return undefined;
// Check if the value of n is null, return the first element of the array if true
if (n == null)
return array[0];
// Check if the value of n is negative, return an empty array if true
if (n < 0)
return [];
// Use the slice method to get the first n elements of the array
return array.slice(0, n);
};
// Testing the function with various cases
console.log(first([7, 9, 0, -2]));
console.log(first([], 3));
console.log(first([7, 9, 0, -2], 3));
console.log(first([7, 9, 0, -2], 6));
console.log(first([7, 9, 0, -2], -3));
Live Demo:
See the Pen JavaScript - Get the first element of an array- array-ex-3 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that returns the first element of an array, and if a number n is provided, returns the first n elements using slice.
- Write a JavaScript function that handles negative n values by returning an empty array.
- Write a JavaScript function that recursively retrieves the first n elements from an array without using built-in methods.
- Write a JavaScript function that validates input and returns a default value when the array is empty.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to clone an array.
Next: Write a JavaScript function to get the last element of an array. Passing a parameter 'n' will return the last 'n' elements of the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.