JavaScript: Check if an array is a factor chain
JavaScript Array: Exercise-51 with Solution
Check Factor Chain
Write a JavaScript program to check if an array is a factor chain or not.
A factor chain is an array in which the previous element is a factor of the next consecutive element. The following is a factor chain:
[2, 4, 8, 16, 32]
// 2 is a factor of 4
// 4 is a factor of 8
// 8 is a factor of 16
// 16 is a factor of 32
Test Data:
([2, 4, 8, 16, 32]) -> true
([2, 4, 16, 32, 64]) -> true
([2, 4, 16, 32, 68]) -> false
Sample Solution:
JavaScript Code :
// Function to check if an array forms a factor chain
function test(nums) {
// Iterate through the array elements up to the second-to-last element
for (var i = 0; i < nums.length - 1; i++)
{
// Check if the next element is not a factor of the current element
if (nums[i + 1] % nums[i] != 0) {
// If not, the array does not form a factor chain
return false;
}
}
// If the loop completes without returning, the array forms a factor chain
return true;
}
// Test the function with an array that forms a factor chain
nums = [2, 4, 8, 16, 32];
console.log("Original array: ", nums);
console.log("Check the said array is a factor chain or not?");
console.log(test(nums));
// Test the function with another array that forms a factor chain
nums = [2, 4, 16, 32, 64];
console.log("Original array: ", nums);
console.log("Check the said array is a factor chain or not?");
console.log(test(nums));
// Test the function with an array that does not form a factor chain
nums = [2, 4, 16, 32, 68];
console.log("Original array: ", nums);
console.log("Check the said array is a factor chain or not?");
console.log(test(nums));
Output:
Original array: Check the said array is a factor chain or not? true Original array: Check the said array is a factor chain or not? true Original array: Check the said array is a factor chain or not? false
Flowchart :
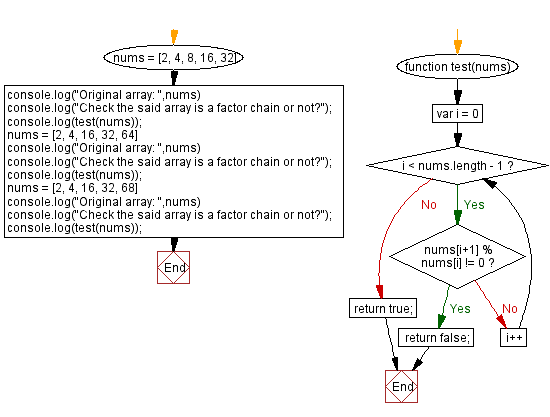
Live Demo :
See the Pen javascript-array-exercise-51 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that verifies if each element in an array is a factor of the next element.
- Write a JavaScript function that uses a loop to check if an array forms a valid factor chain and returns a boolean.
- Write a JavaScript function that validates input and returns true for arrays with a single element.
- Write a JavaScript function that recursively checks the factor chain property without using iterative loops.
Go to:
PREV : Sum Numbers in Mixed Array.
NEXT : Find NaN Indexes in Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.