JavaScript: Find all indexes of NaN in an array
JavaScript Array: Exercise-52 with Solution
Find NaN Indexes in Array
Write a JavaScript program to get all the indexes where NaN is found in a given array of numbers and NaN.
Test Data:
([2, NaN, 8, 16, 32]) -> [1]
([2, 4, NaN, 16, 32, NaN]) -> [2,5]
([2, 4, 16, 32]) ->[]
Sample Solution:
JavaScript Code :
// Function to find all indexes of NaN in an array
function test(arr_nums){
// Use map to create an array of indexes where NaN is found, otherwise return false
return arr_nums.map(function(el, i){
if(isNaN(el))
return i;
return false;
})
// Use filter to remove falsy values (non-NaN elements)
.filter(function(el){
return el;
});
}
// Test the function with an array containing NaN at multiple indexes
arr_nums = [2, NaN, 8, 16, 32];
console.log("Original array: "+arr_nums);
result = test(arr_nums);
console.log("Find all indexes of NaN in the said array: "+result);
// Test the function with another array containing NaN at multiple indexes
arr_nums = [2, 4, NaN, 16, 32, NaN];
console.log("Original array: "+arr_nums);
result = test(arr_nums);
console.log("Find all indexes of NaN in the said array: "+result);
// Test the function with an array without NaN
arr_nums = [2, 4, 16, 32];
console.log("Original array: "+arr_nums);
result = test(arr_nums);
console.log("Find all indexes of NaN in the said array: "+result);
Output:
Original array: 2,NaN,8,16,32 Find all indexes of NaN of the said array: 1 Original array: 2,4,NaN,16,32,NaN Find all indexes of NaN of the said array: 2,5 Original array: 2,4,16,32 Find all indexes of NaN of the said array:
Flowchart :
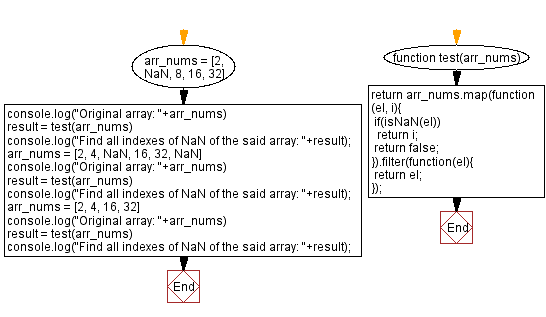
Live Demo :
See the Pen javascript-array-exercise-52 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that iterates over an array and collects all indexes where the element is NaN.
- Write a JavaScript function that uses isNaN() to identify NaN values and returns their positions in an array.
- Write a JavaScript function that properly handles sparse arrays and returns an empty array if no NaN is found.
- Write a JavaScript function that validates the input and returns an array of indices where NaN values occur.
Go to:
PREV : Check Factor Chain.
NEXT : Count Arrays Inside Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.