JavaScript: Calculate multiplication and division of two numbers
JavaScript Basic: Exercise-10 with Solution
Multiplication and Division (User Input)
Write a JavaScript program to calculate multiplication and division of two numbers (input from the user).
Sample Form:
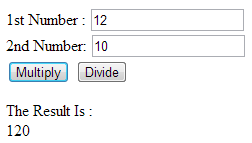
Visual Presentation:
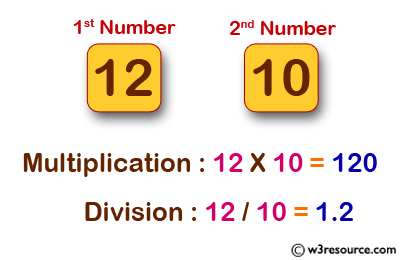
Sample Solution:
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset=utf-8 />
<title>JavaScript program to calculate multiplication and division of two numbers </title>
<style type="text/css">
body {margin: 30px;}
</style>
</head>
<body>
<form>
1st Number : <input type="text" id="firstNumber" /><br>
2nd Number: <input type="text" id="secondNumber" /><br>
<input type="button" onClick="multiplyBy()" Value="Multiply" />
<input type="button" onClick="divideBy()" Value="Divide" />
</form>
<p>The Result is : <br>
<span id = "result"></span>
</p>
</body>
</html>
The JavaScript program prompts the user to input two numbers and then calculates and displays their multiplication and division results. It uses prompt to get the user inputs and basic arithmetic operations to compute the results, which are then logged to the console.
JavaScript Code:
// Define a function to multiply two numbers and display the result
function multiplyBy() {
// Get the values of the input fields with the ids "firstNumber" and "secondNumber"
num1 = document.getElementById("firstNumber").value;
num2 = document.getElementById("secondNumber").value;
// Set the inner HTML of the element with the id "result" to the product of the two numbers
document.getElementById("result").innerHTML = num1 * num2;
}
// Define a function to divide two numbers and display the result
function divideBy() {
// Get the values of the input fields with the ids "firstNumber" and "secondNumber"
num1 = document.getElementById("firstNumber").value;
num2 = document.getElementById("secondNumber").value;
// Set the inner HTML of the element with the id "result" to the quotient of the two numbers
document.getElementById("result").innerHTML = num1 / num2;
}
Live Demo:
See the Pen Javascript: multiplication and division of two numbers - basic - ex-10 by w3resource (@w3resource) on CodePen.
Explanation:
document.getElementById(id).value: The value property sets or returns the value of the value attribute of a text field.
document.getElementById("result").innerHTM : The innerHTML property sets or returns the HTML content (inner HTML) of an element.
ES6 Version:
// Define a function to multiply two numbers and display the result
const multiplyBy = () => {
// Get the value entered in the input field with the id "firstNumber"
const num1 = document.getElementById("firstNumber").value;
// Get the value entered in the input field with the id "secondNumber"
const num2 = document.getElementById("secondNumber").value;
// Set the inner HTML of the element with the id "result" to the product of the two numbers
document.getElementById("result").innerHTML = num1 * num2;
};
// Define a function to divide two numbers and display the result
const divideBy = () => {
// Get the value entered in the input field with the id "firstNumber"
const num1 = document.getElementById("firstNumber").value;
// Get the value entered in the input field with the id "secondNumber"
const num2 = document.getElementById("secondNumber").value;
// Set the inner HTML of the element with the id "result" to the quotient of the two numbers
document.getElementById("result").innerHTML = num1 / num2;
};
For more Practice: Solve these Related Problems:
- Write a JavaScript program that takes two user inputs and returns both the product and quotient rounded to two decimal places.
- Write a JavaScript program that handles division by zero while computing the product and quotient of two user-provided numbers.
- Write a JavaScript program that performs multiplication and division based on user input, returning a custom error message for invalid inputs.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to calculate number of days left until next Christmas.
Next: JavaScript program to convert temperatures to and from celsius, fahrenheit.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.