JavaScript: Calculate number of days left until next Christmas
JavaScript Basic: Exercise-9 with Solution
Days Left Before Christmas
Write a JavaScript program to calculate the days left before Christmas.
The JavaScript program calculates the number of days left until Christmas by comparing the current date with December 25th of the current year. If today is after December 25th, it calculates the days until next year's Christmas. The difference in days is then logged to the console.
Sample Solution:
JavaScript Code:
// Get the current date
today = new Date();
// Create a Date object for Christmas of the current year
var cmas = new Date(today.getFullYear(), 11, 25);
// Check if the current date is after December 25th
if (today.getMonth() == 11 && today.getDate() > 25) {
// If true, set Christmas for the next year
cmas.setFullYear(cmas.getFullYear() + 1);
}
// Calculate the difference in days between today and Christmas
var one_day = 1000 * 60 * 60 * 24;
// Log the number of days left until Christmas to the console
console.log(Math.ceil((cmas.getTime() - today.getTime()) / (one_day)) +
" days left until Christmas!");
Output:
349 days left until Christmas!
Live Demo:
See the Pen JavaScript: days left until next Christmas: basic-ex-9 by w3resource (@w3resource) on CodePen.
Explanation:
Declaring a JavaScript date : In JavaScript Date objects are based on a time value that is the number of milliseconds since 1 January, 1970 UTC. You can declare a date in the following ways :
new Date(); new Date(value); new Date(dateString); new Date(year, month[, day[, hour[, minutes[, seconds[, milliseconds]]]]]);
The getFullYear() method is used to get the year of the specified date according to local time. The value returned by the method is an absolute number. For dates between the years 1000 and 9999, getFullYear() returns a four-digit number, for example, 1985.
The getMonth() method is used to get the month in the specified date according to local time, as a zero-based value. The value returned by getMonth() is an integer between 0 and 11. 0 corresponds to January, 1 to February, and so on.
The getDate() method is used to get the day of the month for the specified date according to local time. The value returned by getDate() is an integer between 1 and 31.
The getTime() method is used to get the numeric value corresponding to the time for the specified date according to universal time.
The Math.ceil() function is used to get the smallest integer greater than or equal to a given number.
Flowchart:
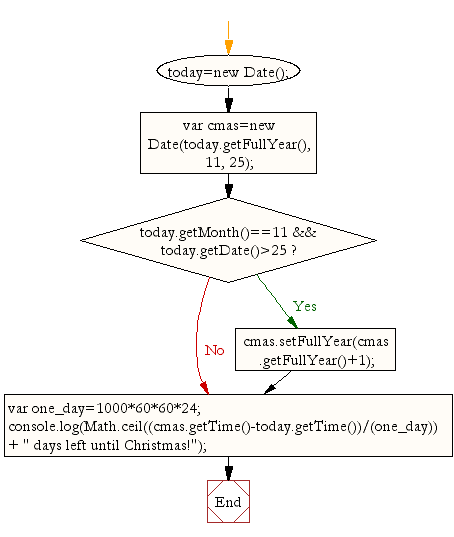
ES6 Version:
// Use the `const` keyword for constants and the `let` keyword for variables
const today = new Date();
const cmas = new Date(today.getFullYear(), 11, 25);
// Check if the current date is after December 25th
if (today.getMonth() === 11 && today.getDate() > 25) {
cmas.setFullYear(cmas.getFullYear() + 1);
}
// Calculate the difference in days and log the result
const one_day = 1000 * 60 * 60 * 24;
console.log(`${Math.ceil((cmas.getTime() - today.getTime()) / one_day)} days left until Christmas!`);
For more Practice: Solve these Related Problems:
- Write a JavaScript program that calculates the number of days left until the next New Year's Eve.
- Write a JavaScript program that displays a live countdown timer for the days left before Christmas.
- Write a JavaScript program that calculates the days left until Christmas, taking into account different time zones.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program where the program takes a random integer between 1 to 10, the user is then prompted to input a guess number.
Next: JavaScript program to calculate multiplication and division of two numbers (input from user).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.