JavaScript: Display a message when a number is between a range
JavaScript Basic: Exercise-8 with Solution
Random Integer Guess Game
Write a JavaScript program where the program takes a random integer between 1 and 10, and the user is then prompted to input a guess number. The program displays a message "Good Work" if the input matches the guess number otherwise "Not matched".
Sample Solution:
JavaScript Code:
// Generate a random integer between 1 and 10 (inclusive)
var num = Math.ceil(Math.random() * 10);
// Log the generated random number to the console
console.log(num);
// Prompt the user to guess a number between 1 and 10 (inclusive)
var gnum = prompt('Guess the number between 1 and 10 inclusive');
// Check if the guessed number matches the generated random number
if (gnum == num)
// Log a message if the guessed number matches the random number
console.log('Matched');
else
// Log a message if the guessed number does not match, and also provide the correct number
console.log('Not matched, the number was ' + gnum);
Output:
Matched
Live Demo:
See the Pen JavaScript basic animation - basic-ex-8 by w3resource (@w3resource) on CodePen.
Explanation:
The Math.ceil() function is used to get the smallest integer greater than or equal to a given number.
The Math.random() function is used to get a floating-point, pseudo-random number in the range [0, 1] that is, from 0 (inclusive) up to but not including 1 (exclusive), which you can then scale to your desired range.
Flowchart:
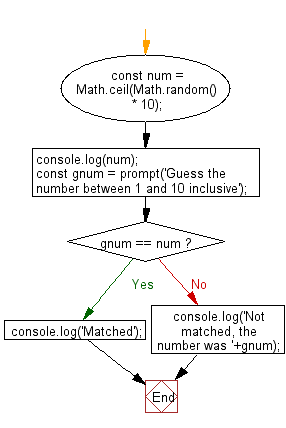
ES6 Version:
// Generate a random integer between 1 and 10 (inclusive)
const num = Math.ceil(Math.random() * 10);
// Log the generated random number to the console
console.log(num);
// Prompt the user to guess a number between 1 and 10 (inclusive)
const gnum = prompt('Guess the number between 1 and 10 inclusive');
// Check if the guessed number matches the generated random number
if (gnum == num) {
// Log a message if the guessed number matches the random number
console.log('Matched');
} else {
// Log a message if the guessed number does not match, and also provide the correct number
console.log('Not matched, the number was ' + gnum);
}
For more Practice: Solve these Related Problems:
- Write a JavaScript program that implements a random integer guess game with a limited number of attempts.
- Write a JavaScript program that provides hints to the user if the guessed number is too high or too low.
- Write a JavaScript program for a random integer guess game that tracks and displays the number of attempts before a correct guess.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to find which 1st January is being a Sunday between 2014 and 2050.
Next: JavaScript program to calculate number of days left until next Christmas.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.