JavaScript: Find 1st January be a Sunday between a range of years
JavaScript Basic: Exercise-7 with Solution
Write a JavaScript program to find out if 1st January will be a Sunday between 2014 and 2050.
To find out which years between 2014 and 2050 have 1st January falling on a Sunday, we can use the following approach:
- Loop through each year between 2014 and 2050 and check if the 1st January of that year falls on a Sunday.
- To check if the 1st January of a year is a Sunday, we can use the Date object in JavaScript. Create a Date object for the 1st January of each year and use the getDay() method to get the day of the week. The getDay() method returns an integer between 0 and 6, where 0 represents Sunday, 1 represents Monday, and so on.
- If the day of the week is 0 (Sunday), we can print out the year.
Here is the algorithm in pseudocode:
for year = 2014 to 2050 do create a Date object for 1st January of year if day of the week of Date object is Sunday then print year end if end for
Using this approach, we can find out that the years with 1st January falling on a Sunday between 2014 and 2050.
Sample Solution:
JavaScript Code:
// Log a separator to visually distinguish the output
console.log('--------------------');
// Loop through the years from 2014 to 2050 (inclusive)
for (var year = 2014; year <= 2050; year++) {
// Create a Date object for January 1st of the current year
var d = new Date(year, 0, 1);
// Check if January 1st is a Sunday (where Sunday corresponds to day index 0)
if (d.getDay() === 0) {
// Log a message if January 1st is a Sunday for the current year
console.log("1st January is being a Sunday " + year);
}
}
// Log another separator to conclude the output
console.log('--------------------');
Output:
-------------------- 1st January is being a Sunday 2017 1st January is being a Sunday 2023 1st January is being a Sunday 2034 1st January is being a Sunday 2040 1st January is being a Sunday 2045 --------------------
Live Demo:
See the Pen JavaScript: Sunday 2014 and 2050 - basic-ex-7 by w3resource (@w3resource) on CodePen.
Explanation:
Declaring a JavaScript date : In JavaScript Date objects are based on a time value that is the number of milliseconds since 1 January, 1970 UTC. You can declare a date in the following ways :
new Date(); new Date(value); new Date(dateString); new Date(year, month[, day[, hour[, minutes[, seconds[, milliseconds]]]]]);
The getDay() method is used to get the day of the week for the specified date according to local time, where 0 represents Sunday. The value returned by getDay() is an integer corresponding to the day of the week: 0 for Sunday, 1 for Monday, 2 for Tuesday, and so on.
Flowchart:
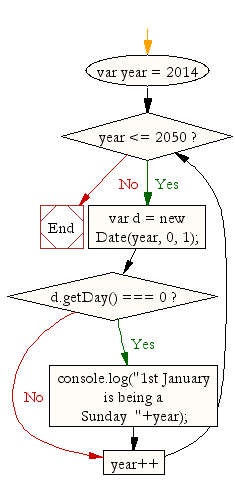
ES6 Version:
// Log a separator to visually distinguish the output
console.log('--------------------');
// Loop through the years from 2014 to 2050 (inclusive)
for (let year = 2014; year <= 2050; year++) {
// Create a Date object for January 1st of the current year
const d = new Date(year, 0, 1);
// Check if January 1st is a Sunday (where Sunday corresponds to day index 0)
if (d.getDay() === 0) {
// Log a message if January 1st is a Sunday for the current year using template literals
console.log(`1st January is being a Sunday ${year}`);
}
}
// Log another separator to conclude the output
console.log('--------------------');
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to determine whether a given year is a leap year in the Gregorian calendar.
Next: JavaScript program where the program takes a random integer between 1 to 10, the user is then prompted to input a guess number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics