JavaScript: Check whether a given string represents a correct sentence or not
JavaScript Basic: Exercise-114 with Solution
Check String as Correct Sentence
Write a JavaScript program to check whether a given string represents a correct sentence or not. A string is considered a correct sentence if it starts with a capital letter and ends with a full stop (.).
Sample Solution:
JavaScript Code:
// Check if the input string is a correct sentence based on certain conditions
function is_correct_Sentence(input_str) {
var first_char = input_str[0]; // Get the first character of the string
var last_char = input_str[input_str.length - 1]; // Get the last character of the string
return /[A-Z]/.test(first_char) && last_char == "."; // Check if the first character is an uppercase letter and the last character is a period.
}
// Examples of checking correct sentences using the function
console.log(is_correct_Sentence("This tool will help you write better English and efficiently corrects texts.")); // Output: true (It's a correct sentence)
console.log(is_correct_Sentence("This tool will help you write better English and efficiently corrects texts")); // Output: false (Missing period at the end)
console.log(is_correct_Sentence("this tool will help you write better English and efficiently corrects texts.")); // Output: false (First letter should be capitalized)
Output:
true false false
Live Demo:
See the Pen javascript-basic-exercise-114 by w3resource (@w3resource) on CodePen.
Flowchart:
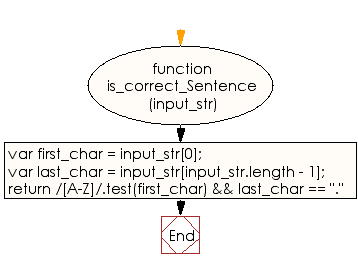
ES6 Version:
// Check if the input string is a correct sentence based on certain conditions
const is_correct_Sentence = (input_str) => {
// Get the first character of the string
const first_char = input_str[0];
// Get the last character of the string
const last_char = input_str[input_str.length - 1];
// Check if the first character is an uppercase letter and the last character is a period
return /[A-Z]/.test(first_char) && last_char === ".";
};
// Examples of checking correct sentences using the function
console.log(is_correct_Sentence("This tool will help you write better English and efficiently corrects texts.")); // Output: true (It's a correct sentence)
console.log(is_correct_Sentence("This tool will help you write better English and efficiently corrects texts")); // Output: false (Missing period at the end)
console.log(is_correct_Sentence("this tool will help you write better English and efficiently corrects texts.")); // Output: false (First letter should be capitalized)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if a string starts with an uppercase letter and ends with a period after trimming whitespace.
- Write a JavaScript function that validates sentence structure by ensuring proper capitalization and punctuation.
- Write a JavaScript program that verifies whether a given string qualifies as a correct sentence based on initial capital and ending full stop criteria.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to calculate the sum n + n/2 + n/4 + n/8 + .... where n is a positive integer and all divisions are integer.
Next: JavaScript program to check whether a matrix is a diagonal matrix or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.