JavaScript: Check whether a matrix is a diagonal matrix or not
JavaScript Basic: Exercise-115 with Solution
Check if Matrix is Diagonal Matrix
Write a JavaScript program to check whether a matrix is a diagonal matrix or not. In linear algebra, a diagonal matrix is a matrix in which the entries outside the main diagonal are all zero (the diagonal from the upper left to the lower right).
Example:
[1, 0, 0], [0, 2, 0], [0, 0, 3] ]) = true
[1, 0, 0], [0, 2, 3], [0, 0, 3] ]) = false
Visual Presentation:
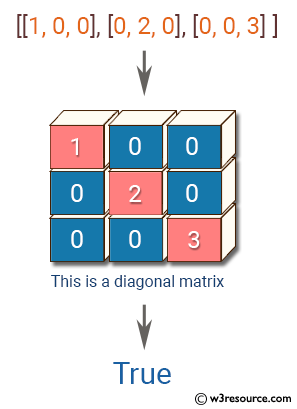
Sample Solution:
JavaScript Code:
// Function to check if a matrix is a diagonal matrix
const is_diagonal_matrix = (user_matrix) => {
// Loop through each row of the matrix
for (let i = 0; i < user_matrix.length; i++) {
// Loop through each element in the row
for (let j = 0; j < user_matrix.length; j++) {
// Check if the element is non-zero when it's not on the diagonal (i !== j)
if (i !== j && user_matrix[i][j] !== 0)
return false; // Return false if non-zero value is found off the diagonal
}
}
return true; // Return true if all non-diagonal elements are zero
}
// Testing the function with sample matrix inputs
console.log(is_diagonal_matrix([[1, 0, 0], [0, 2, 0], [0, 0, 3]])); // Output: true (Diagonal matrix)
console.log(is_diagonal_matrix([[1, 0, 0], [0, 2, 3], [0, 0, 3]])); // Output: false (Non-diagonal elements present)
Output:
true false
Live Demo:
See the Pen javascript-basic-exercise-115 by w3resource (@w3resource) on CodePen.
Flowchart:
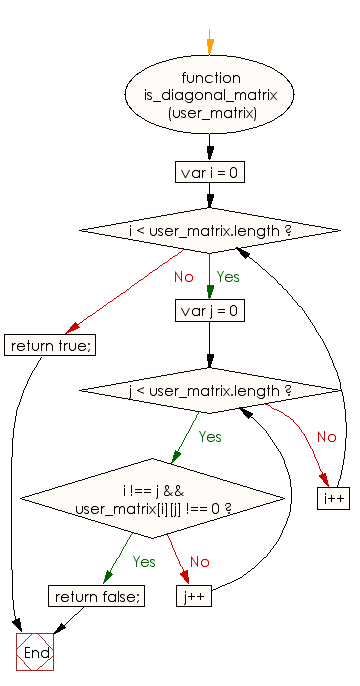
ES6 Version:
// Function to check if a matrix is a diagonal matrix
const is_diagonal_matrix = (user_matrix) => {
// Loop through each row of the matrix
for (let i = 0; i < user_matrix.length; i++) {
// Loop through each element in the row
for (let j = 0; j < user_matrix.length; j++) {
// Check if the element is non-zero when it's not on the diagonal (i !== j)
if (i !== j && user_matrix[i][j] !== 0) {
return false; // Return false if non-zero value is found off the diagonal
}
}
}
return true; // Return true if all non-diagonal elements are zero
}
// Testing the function with sample matrix inputs
console.log(is_diagonal_matrix([[1, 0, 0], [0, 2, 0], [0, 0, 3]])); // Output: true (Diagonal matrix)
console.log(is_diagonal_matrix([[1, 0, 0], [0, 2, 3], [0, 0, 3]])); // Output: false (Non-diagonal elements present)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if a square matrix is diagonal by confirming that all off-diagonal elements are zero.
- Write a JavaScript function that iterates through a matrix and returns true only if non-diagonal entries are all zero.
- Write a JavaScript program that validates a matrix as diagonal by comparing each element's row and column indices.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether a given string represents a correct sentence or not.
Next: JavaScript program to find all the possible options to replace the hash in a string (Consists of digits and one hash (#)) with a digit to produce an integer divisible by 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.