JavaScript: Check whether a given matrix is an identity matrix
JavaScript Basic: Exercise-117 with Solution
Check if Matrix is Identity Matrix
Write a JavaScript program to check whether a given matrix is an identity matrix.
Note: In linear algebra, the identity matrix, or sometimes ambiguously called a unit matrix, of size n is the n × n square matrix with ones on the main diagonal and zeros elsewhere.
[[1, 0, 0], [0, 1, 0], [0, 0, 1]] -> true
[[1, 0, 0], [0, 1, 0], [1, 0, 1]] -> false
Visual Presentation:
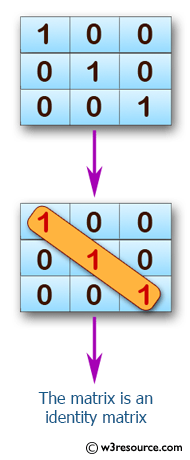
Sample Solution:
JavaScript Code:
// Function to check if the given matrix is an identity matrix
function is_identity_Matrix(matrix_data) {
// Checks whether given matrix is a square matrix or not
for (var i = 0; i < matrix_data.length; i++) {
rows = matrix_data.length;
cols = matrix_data[i].length;
if (rows != cols) {
console.log("Matrix should be a square matrix");
return false;
}
}
// Loop to verify if the matrix is an identity matrix
for (var i = 0; i < matrix_data.length; i++) {
for (var j = 0; j < matrix_data.length; j++) {
if (matrix_data[i][j] !== 1 && i === j || matrix_data[i][j] && i !== j) {
return false;
}
}
}
return true; // Returns true if the matrix is an identity matrix
}
// Testing the function with sample inputs
console.log(is_identity_Matrix([[1, 0, 0, 2], [0, 1, 0], [0, 0, 1]])); // Output: false (not a square matrix)
console.log(is_identity_Matrix([[1, 0, 0], [0, 1, 0], [0, 0, 1]])); // Output: true (identity matrix)
console.log(is_identity_Matrix([[1, 0, 1], [0, 1, 0], [0, 0, 1]])); // Output: false (not an identity matrix)
Output:
Matrix should be a square matrix false true false
Live Demo:
See the Pen javascript-basic-exercise-117 by w3resource (@w3resource) on CodePen.
Flowchart:
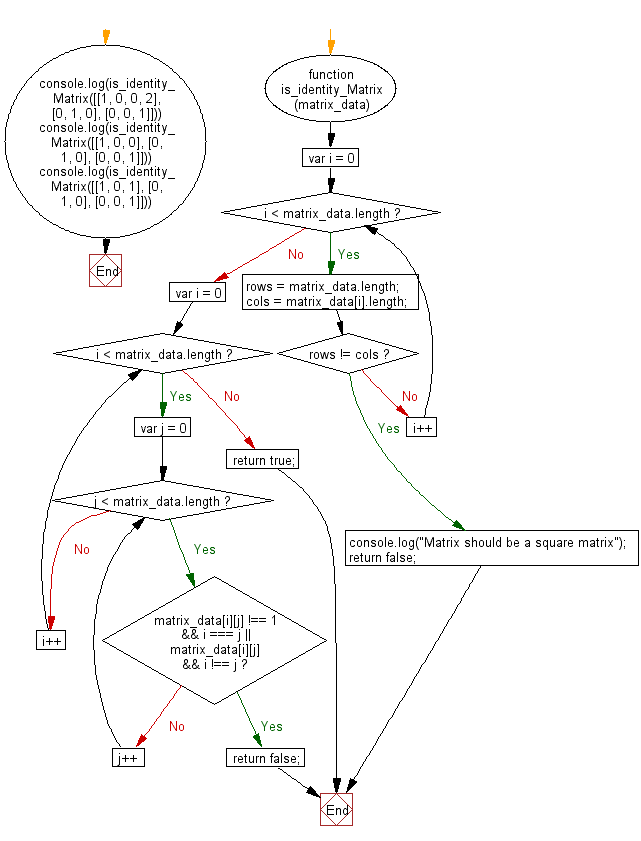
ES6 Version:
// Function to check if the given matrix is an identity matrix
const is_identity_Matrix = (matrix_data) => {
// Checks whether the given matrix is a square matrix
for (let i = 0; i < matrix_data.length; i++) {
const rows = matrix_data.length;
const cols = matrix_data[i].length;
if (rows !== cols) {
console.log("Matrix should be a square matrix");
return false;
}
}
// Verifies if the matrix is an identity matrix
for (let i = 0; i < matrix_data.length; i++) {
for (let j = 0; j < matrix_data.length; j++) {
if ((matrix_data[i][j] !== 1 && i === j) || (matrix_data[i][j] && i !== j)) {
return false;
}
}
}
return true; // Returns true if the matrix is an identity matrix
};
// Testing the function with sample inputs
console.log(is_identity_Matrix([[1, 0, 0, 2], [0, 1, 0], [0, 0, 1]])); // Output: false (not a square matrix)
console.log(is_identity_Matrix([[1, 0, 0], [0, 1, 0], [0, 0, 1]])); // Output: true (identity matrix)
console.log(is_identity_Matrix([[1, 0, 1], [0, 1, 0], [0, 0, 1]])); // Output: false (not an identity matrix)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that verifies whether a given square matrix is an identity matrix by checking that diagonal elements are 1 and others 0.
- Write a JavaScript function that iterates through a matrix and returns true only if it meets identity matrix criteria.
- Write a JavaScript program that compares each element of a matrix against the identity matrix properties, handling non-square inputs gracefully.
Go to:
PREV : Replace Hash in String to Make Divisible by 3.
NEXT : Check if Number is in Range.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.