JavaScript: Check whether a given number is in a given range
JavaScript Basic: Exercise-118 with Solution
Check if Number is in Range
Write a JavaScript program to check whether a given number is in a given range.
Visual Presentation:
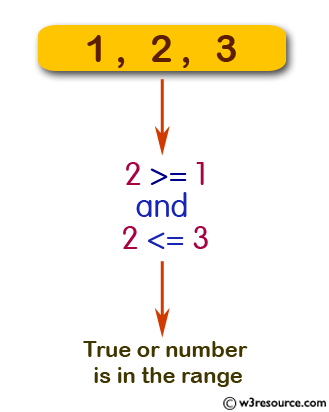
Sample Solution:
JavaScript Code:
// Function to check if 'y' lies within the range of 'x' and 'z'
function is_inrange(x, y, z)
{
return y >= x && y <= z; // Returns true if 'y' is between 'x' and 'z' (inclusive)
}
console.log(is_inrange(1,2,3)); // Output: true (2 is in the range between 1 and 3)
console.log(is_inrange(1,2,-3)); // Output: false (2 is not in the range between 1 and -3)
console.log(is_inrange(1.1,1.2,1.3)); // Output: true (1.2 is in the range between 1.1 and 1.3)
Output:
true false true
Live Demo:
See the Pen javascript-basic-exercise-118 by w3resource (@w3resource) on CodePen.
Flowchart:
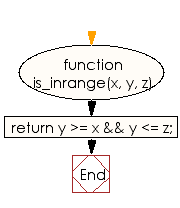
ES6 Version:
// Function to check if 'y' lies within the range of 'x' and 'z'
const is_inrange = (x, y, z) => {
return y >= x && y <= z; // Returns true if 'y' is between 'x' and 'z' (inclusive)
};
console.log(is_inrange(1,2,3)); // Output: true (2 is in the range between 1 and 3)
console.log(is_inrange(1,2,-3)); // Output: false (2 is not in the range between 1 and -3)
console.log(is_inrange(1.1,1.2,1.3)); // Output: true (1.2 is in the range between 1.1 and 1.3)
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check whether a given matrix is an identity matrix.
Next: JavaScript program to check whether a given integer has an increasing digits sequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-basic-exercise-118.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics