JavaScript: Create the value of NOR of two given booleans
JavaScript Basic: Exercise-124 with Solution
Compute NOR of Two Booleans
Write a JavaScript program to create the NOR value of two given booleans.
Note: In boolean logic, logical nor or joint denial is a truth-functional operator which produces a result that is the negation of logical or. That is, a sentence of the form (p NOR q) is true precisely when neither p nor q is true - i.e. when both of p and q are false
Sample Example:
For x = true and y = false, the output should be logical_Nor(x, y) = false; For x = false and y = false, the output should be logical_Nor(x, y) = true.
Sample Solution:
JavaScript Code:
// Function to perform logical NOR operation
function test_logical_Nor(a, b) {
return (!a && !b); // Returns true only if both a and b are false
}
// Testing logical NOR operation with different input values
console.log(test_logical_Nor(true, false)); // Output: false (logical NOR of true and false is false)
console.log(test_logical_Nor(false, false)); // Output: true (logical NOR of false and false is true)
console.log(test_logical_Nor(true, true)); // Output: false (logical NOR of true and true is false)
Output:
false true false
Live Demo:
See the Pen javascript-basic-exercise-124 by w3resource (@w3resource) on CodePen.
Flowchart:
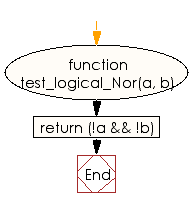
ES6 Version:
// ES6 function to perform logical NOR operation
const test_logical_Nor = (a, b) => {
return (!a && !b); // Returns true only if both a and b are false
};
// Testing logical NOR operation with different input values
console.log(test_logical_Nor(true, false)); // Output: false (logical NOR of true and false is false)
console.log(test_logical_Nor(false, false)); // Output: true (logical NOR of false and false is true)
console.log(test_logical_Nor(true, true)); // Output: false (logical NOR of true and true is false)
For more Practice: Solve these Related Problems:
- Write a JavaScript program that computes the NOR of two boolean values by first calculating their logical OR and then negating the result.
- Write a JavaScript function that takes two boolean inputs and returns true only if both are false, simulating the NOR operation.
- Write a JavaScript program that implements the NOR logic gate by combining NOT and OR operators on two boolean values.
Go to:
PREV : Check if Array is Permutation of Numbers 1 to n.
NEXT : Find Longest String in Array.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.