JavaScript: Find the smallest round number that is not less than a given value
JavaScript Basic: Exercise-128 with Solution
Find Smallest Round Number ≥ Value
Write a JavaScript program to find the smallest round number not less than a given value.
Note: A round number is informally considered to be an integer that ends with one or more zeros. So, 590 is rounder than 592, but 590 is less round than 600.
Visual Presentation:
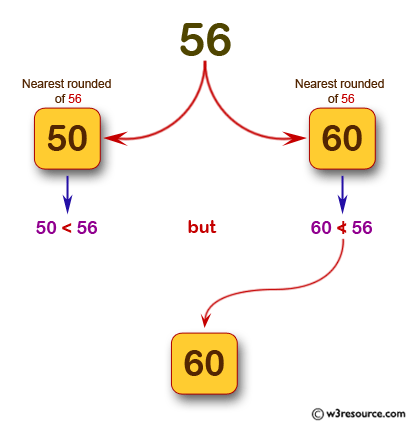
Sample Solution:
JavaScript Code:
// Function to find the nearest round number
function nearest_round_number(num) {
// Loop until the number is divisible by 10
while (num % 10) {
// Increment the number by 1 until it is divisible by 10
num++;
}
// Return the nearest round number
return num;
}
// Test cases
console.log(nearest_round_number(56)); // Output: 60
console.log(nearest_round_number(592)); // Output: 600
Output:
60 600
Live Demo:
See the Pen javascript-basic-exercise-128 by w3resource (@w3resource) on CodePen.
Flowchart:
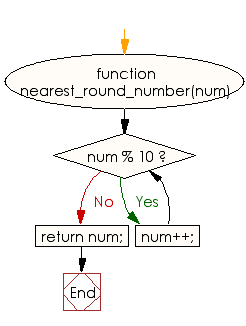
ES6 Version:
// Function to find the nearest round number
const nearest_round_number = (num) => {
// Loop until the number is divisible by 10
while (num % 10) {
// Increment the number by 1 until it is divisible by 10
num++;
}
// Return the nearest round number
return num;
};
// Test cases
console.log(nearest_round_number(56)); // Output: 60
console.log(nearest_round_number(592)); // Output: 600
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds the smallest round number (ending with one or more zeros) that is not less than a given value.
- Write a JavaScript function that increments a number until a round number is reached, handling edge cases appropriately.
- Write a JavaScript program that calculates the next round number by adjusting the least significant digits to zero based on the input.
Go to:
PREV : Reverse Bits in Integer.
NEXT : Find Smallest Prime > Value.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.