JavaScript: Sort the strings of a given array of strings in the order of increasing lengths
JavaScript Basic: Exercise-143 with Solution
Sort Strings by Increasing Length
Write a JavaScript program to sort the strings of a given array of strings in order of increasing length.
Note: Do not change the order if the lengths of two string are same.
Visual Presentation:
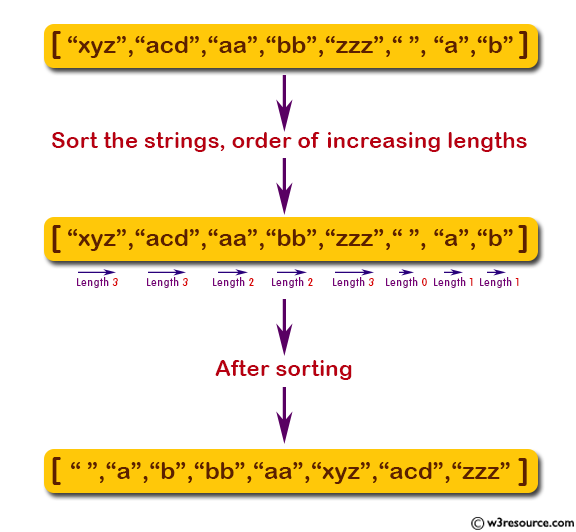
Sample Solution:
JavaScript Code:
/**
* Function to sort an array of strings based on string length
* @param {array} arra - The array of strings to be sorted
* @returns {array} - The sorted array of strings
*/
function sort_by_string_length(arra) {
// Loop through each element in the array
for (var i = 0; i < arra.length; i++) {
// Compare the current element with the subsequent elements
for (var j = i + 1; j < arra.length; j++) {
// If the length of the current element is greater than the subsequent element
if (arra[i].length > arra[j].length) {
var m = arra[i]; // Store the current element in a temporary variable
arra[i] = arra[j]; // Swap the current element with the subsequent element
arra[j] = m; // Place the temporary variable (previous value of current element) in the subsequent element
}
}
}
return arra; // Return the sorted array
}
var arra = ["xyz", "acd", "aa", "bb", "zzz", "", "a", "b"]; // Input array
console.log("Original array: " + arra + "\n"); // Display original array
console.log(sort_by_string_length(["xyz", "acd", "aa", "bb", "zzz", "", "a", "b"])); // Sort and display the array
Output:
Original array: xyz,acd,aa,bb,zzz,,a,b ["","a","b","bb","aa","xyz","acd","zzz"]
Live Demo:
See the Pen javascript-basic-exercise-143 by w3resource (@w3resource) on CodePen.
Flowchart:
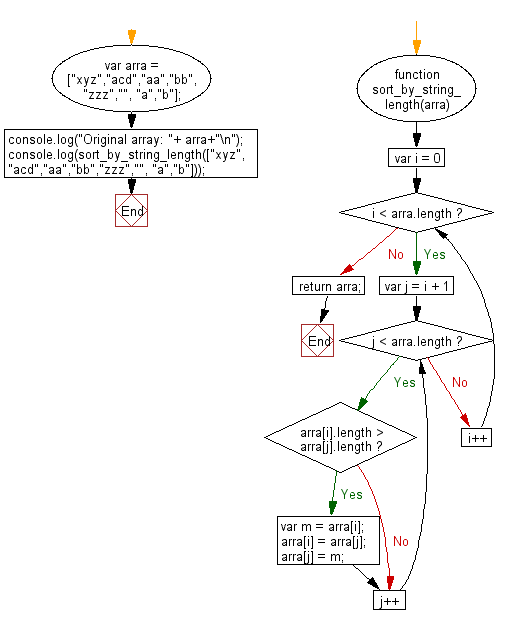
ES6 Version:
/**
* Function to sort an array of strings based on string length
* @param {array} arra - The array of strings to be sorted
* @returns {array} - The sorted array of strings
*/
const sort_by_string_length = (arra) => {
// Loop through each element in the array
for (let i = 0; i < arra.length; i++) {
// Compare the current element with the subsequent elements
for (let j = i + 1; j < arra.length; j++) {
// If the length of the current element is greater than the subsequent element
if (arra[i].length > arra[j].length) {
// Swap the current element with the subsequent element using destructuring assignment
[arra[i], arra[j]] = [arra[j], arra[i]];
}
}
}
return arra; // Return the sorted array
};
// Input array
const arra = ["xyz", "acd", "aa", "bb", "zzz", "", "a", "b"];
// Display original array
console.log("Original array: ", arra, "\n");
// Sort and display the array
console.log(sort_by_string_length(["xyz", "acd", "aa", "bb", "zzz", "", "a", "b"]));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that sorts an array of strings by increasing length while preserving the order of strings with equal lengths.
- Write a JavaScript function that orders strings based on their length using a stable sorting algorithm.
- Write a JavaScript program that rearranges an array of strings into ascending order by length, ensuring the original order is maintained for same-length strings.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to simplify a given absolute path for a file in Unix-style.
Next: JavaScript program to break an address of an url and put it's part into an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.