JavaScript: Create a new string from a given string changing the position of first and last characters
JavaScript Basic: Exercise-23 with Solution
Swap First and Last Characters in String
Write a JavaScript program to create a new string from a given string by changing the position of the first and last characters. The string length must be broader than or equal to 1.
This JavaScript program creates a new string from a given string by swapping the positions of the first and last characters. It ensures that the length of the string is greater than or equal to 1 to perform the swap operation safely. By using string manipulation methods like charAt() and concatenation, it constructs the new string with the first and last characters swapped.
Visual Presentation:
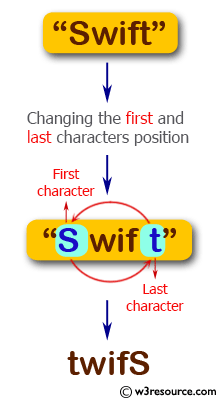
Sample Solution:
JavaScript Code:
// Define a function named first_last that takes a parameter str1
function first_last(str1) {
// Check if the length of str1 is less than or equal to 1
if (str1.length <= 1) {
// If true, return str1 as is
return str1;
}
// Extract the substring from the second character to the second-to-last character of str1
mid_char = str1.substring(1, str1.length - 1);
// Return the last character of str1 followed by mid_char and then the first character of str1
return (str1.charAt(str1.length - 1)) + mid_char + str1.charAt(0);
}
// Log the result of calling the first_last function with the argument 'a' to the console
console.log(first_last('a'));
// Log the result of calling the first_last function with the argument 'ab' to the console
console.log(first_last('ab'));
// Log the result of calling the first_last function with the argument 'abc' to the console
console.log(first_last('abc'));
Output:
a ba cba
Live Demo:
See the Pen JavaScript: position of first and last characters.: basic-ex-23 by w3resource (@w3resource) on CodePen.
Flowchart:
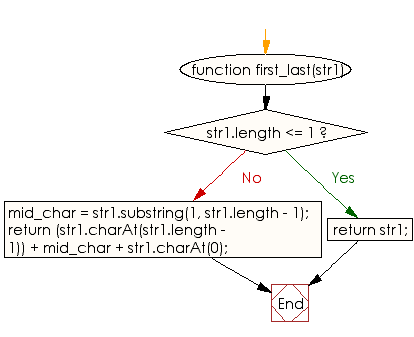
ES6 Version:
// Using ES6 arrow function syntax to define the first_last function
const first_last = (str1) => {
// Check if the length of str1 is less than or equal to 1
if (str1.length <= 1) {
// If true, return str1 as is
return str1;
}
// Extract the substring from the second character to the second-to-last character of str1
const mid_char = str1.substring(1, str1.length - 1);
// Return the last character of str1 followed by mid_char and then the first character of str1
return (str1.charAt(str1.length - 1)) + mid_char + str1.charAt(0);
};
// Log the result of calling the first_last function with the argument 'a' to the console
console.log(first_last('a'));
// Log the result of calling the first_last function with the argument 'ab' to the console
console.log(first_last('ab'));
// Log the result of calling the first_last function with the argument 'abc' to the console
console.log(first_last('abc'));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to remove a character at the specified position of a given string and return the new string.
Next: JavaScript program to create a new string from a given string with the first character of the given string added at the front and back.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-basic-exercise-23.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics