JavaScript: Create a new string from a given string with the first character of the given string added at the front and back
JavaScript Basic: Exercise-24 with Solution
Add First Character to Front and Back of String
Write a JavaScript program to create another string from a given string with the first character of the given string added to the front and back.
This JavaScript program creates a new string by adding the first character of the given string to both the front and back. It retrieves the first character using charAt(0) or substring() function and then concatenates it with the original string twice, once at the beginning and once at the end, to form the new string.
Visual Presentation:
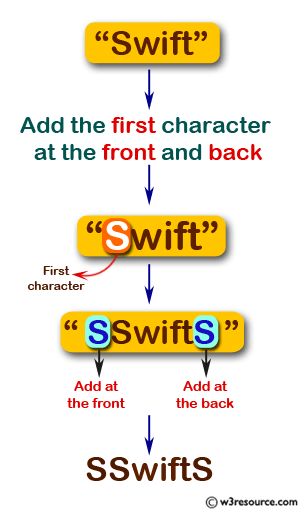
Sample Solution:
JavaScript Code:
// Define a function named front_back that takes a parameter str
function front_back(str) {
// Extract the first character of str
first = str.substring(0, 1);
// Return the concatenation of first, str, and first
return first + str + first;
}
// Log the result of calling the front_back function with the argument 'a' to the console
console.log(front_back('a'));
// Log the result of calling the front_back function with the argument 'ab' to the console
console.log(front_back('ab'));
// Log the result of calling the front_back function with the argument 'abc' to the console
console.log(front_back('abc'));
Output:
aaa aaba aabca
Live Demo:
See the Pen JavaScript: string added at the front and back: basic-ex-24 by w3resource (@w3resource) on CodePen.
Flowchart:
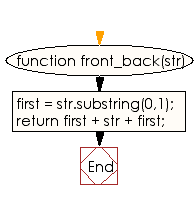
ES6 Version:
// Using ES6 arrow function syntax to define the front_back function
const front_back = (str) => {
// Extract the first character of str
const first = str.substring(0, 1);
// Return the concatenation of first, str, and first
return first + str + first;
};
// Log the result of calling the front_back function with the argument 'a' to the console
console.log(front_back('a'));
// Log the result of calling the front_back function with the argument 'ab' to the console
console.log(front_back('ab'));
// Log the result of calling the front_back function with the argument 'abc' to the console
console.log(front_back('abc'));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that adds the first character of a string to both the beginning and end, unless the string has only one character.
- Write a JavaScript program that prepends and appends the first character of a string, then duplicates the entire string.
- Write a JavaScript program that adds the first character to the front and back of the string, and then reverses the rest of the string.
Go to:
PREV : Swap First and Last Characters in String.
NEXT : Check if Number is Multiple of 3 or 7.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.