JavaScript: Find a value which is nearest to 100 from two different given integer values
JavaScript Basic: Exercise-32 with Solution
Find Closest Value to 100 from Two Numbers
Write a JavaScript program to find the closest value to 100 from two numerical values.
This JavaScript program compares two numerical values and determines which one is closest to 100. It calculates the absolute difference between each value and 100, then returns the value with the smaller difference.
Visual Presentation - 1:
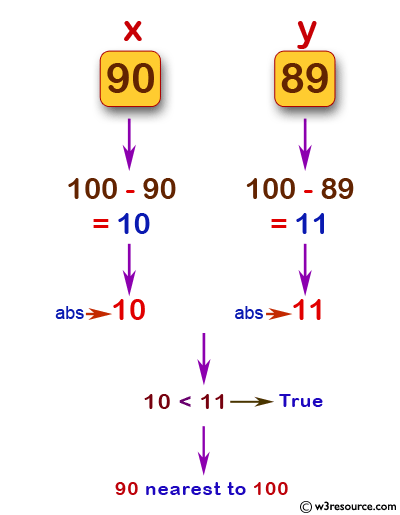
Visual Presentation - 2:
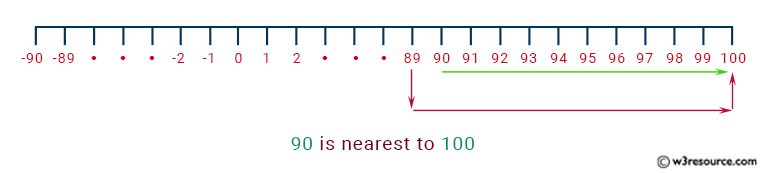
Sample Solution:
JavaScript Code:
// Define a function named near_100 with parameters x and y
function near_100(x, y) {
// Check if x is not equal to y
if (x != y) {
// Calculate the absolute difference between x and 100, store it in x1
x1 = Math.abs(x - 100);
// Calculate the absolute difference between y and 100, store it in y1
y1 = Math.abs(y - 100);
// Compare x1 and y1 to determine which value is closer to 100
if (x1 < y1) {
// Return x if x is closer to 100
return x;
}
// Return y if y is closer to 100
if (y1 < x1) {
return y;
}
// Return 0 if x and y are equidistant from 100
return 0;
} else {
// Return false if x is equal to y
return false;
}
}
// Log the result of calling near_100 with the arguments 90 and 89 to the console
console.log(near_100(90, 89));
// Log the result of calling near_100 with the arguments -90 and -89 to the console
console.log(near_100(-90, -89));
// Log the result of calling near_100 with the arguments 90 and 90 to the console
console.log(near_100(90, 90));
Output:
90 -89 false
Live Demo:
See the Pen JavaScript: value which is nearest to 100 - ex-32 by w3resource (@w3resource) on CodePen.
Flowchart:
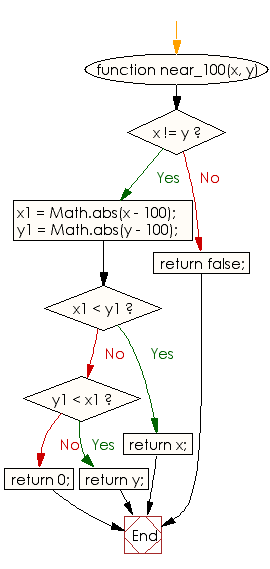
ES6 Version:
// Define a function named near_100 with parameters x and y
const near_100 = (x, y) => {
// Check if x is not equal to y
if (x !== y) {
// Calculate the absolute difference between x and 100, store it in x1
const x1 = Math.abs(x - 100);
// Calculate the absolute difference between y and 100, store it in y1
const y1 = Math.abs(y - 100);
// Compare x1 and y1 to determine which value is closer to 100
if (x1 < y1) {
// Return x if x is closer to 100
return x;
}
// Return y if y is closer to 100
if (y1 < x1) {
return y;
}
// Return 0 if x and y are equidistant from 100
return 0;
} else {
// Return false if x is equal to y
return false;
}
};
// Log the result of calling near_100 with the arguments 90 and 89 to the console
console.log(near_100(90, 89));
// Log the result of calling near_100 with the arguments -90 and -89 to the console
console.log(near_100(-90, -89));
// Log the result of calling near_100 with the arguments 90 and 90 to the console
console.log(near_100(90, 90));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that compares two numbers and returns the one closest to 100, handling ties by returning both values.
- Write a JavaScript program that determines which of two numbers is closer to 100 and returns a message if they are equidistant.
- Write a JavaScript program that takes two numbers and identifies the one with the smallest absolute difference from 100.
Go to:
PREV : Find Largest of Three Integers.
NEXT : Check if Two Numbers are in Specific Ranges.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.