JavaScript: Find the largest of three given integers
JavaScript Basic: Exercise-31 with Solution
Find Largest of Three Integers
Write a JavaScript program to find the largest of three given integers.
This JavaScript program takes three integers as input and determines the largest among them. It compares the integers using conditional statements and returns the highest value.
Visual Presentation:
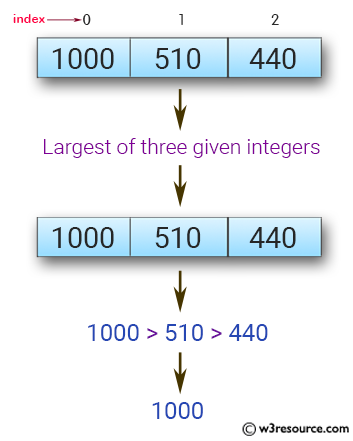
Sample Solution:
JavaScript Code:
// Define a function named max_of_three that takes three parameters: x, y, and z
function max_of_three(x, y, z) {
// Initialize a variable max_val with the value 0
let max_val = 0;
// Check if x is greater than y
if (x > y) {
// If true, assign the value of x to max_val
max_val = x;
} else {
// If false, assign the value of y to max_val
max_val = y;
}
// Check if z is greater than max_val
if (z > max_val) {
// If true, update max_val to the value of z
max_val = z;
}
// Return the final value of max_val
return max_val;
}
// Log the result of calling max_of_three with the arguments 1, 0, 1 to the console
console.log(max_of_three(1, 0, 1));
// Log the result of calling max_of_three with the arguments 0, -10, -20 to the console
console.log(max_of_three(0, -10, -20));
// Log the result of calling max_of_three with the arguments 1000, 510, 440 to the console
console.log(max_of_three(1000, 510, 440));
Output:
1 0 1000
Live Demo:
See the Pen JavaScript: largest of three given integers - ex-31 by w3resource (@w3resource) on CodePen.
Flowchart:
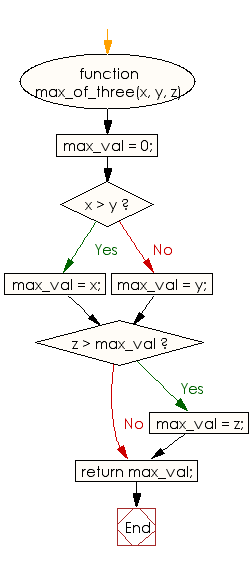
ES6 Version:
// Define a function named max_of_three using arrow function syntax
const max_of_three = (x, y, z) => {
// Initialize a variable max_val with the value 0
let max_val = 0;
// Use the conditional (ternary) operator to assign the maximum value between x and y to max_val
max_val = (x > y) ? x : y;
// Update max_val to the value of z if z is greater than max_val
max_val = (z > max_val) ? z : max_val;
// Return the final value of max_val
return max_val;
};
// Log the result of calling max_of_three with the arguments 1, 0, 1 to the console
console.log(max_of_three(1, 0, 1));
// Log the result of calling max_of_three with the arguments 0, -10, -20 to the console
console.log(max_of_three(0, -10, -20));
// Log the result of calling max_of_three with the arguments 1000, 510, 440 to the console
console.log(max_of_three(1000, 510, 440));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds the largest among three integers without using Math.max().
- Write a JavaScript program that determines the largest of three numbers, including negative values.
- Write a JavaScript program that accepts three integers and returns the largest using a nested ternary operator.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to check if a string "Script" presents at 5th (index 4) position in a given string,
Next: JavaScript program to find a value which is nearest to 100 from two different given integer values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.