JavaScript: Find the area of a triangle where lengths of the three of its sides are 5, 6, 7
JavaScript Basic: Exercise-4 with Solution
Calculate Area of Triangle (Sides: 5, 6, 7)
Write a JavaScript program to find the area of a triangle where three sides are 5, 6, 7.
This JavaScript program calculates the area of a triangle with sides of lengths 5, 6, and 7 using Heron's formula. It first computes the semi-perimeter (s) of the triangle, then uses it to find the area, which is logged to the console.
Sample Solution:
JavaScript Code:
// Define the lengths of the three sides of a triangle
var side1 = 5;
var side2 = 6;
var side3 = 7;
// Calculate the semi-perimeter of the triangle
var s = (side1 + side2 + side3) / 2;
// Use Heron's formula to calculate the area of the triangle
var area = Math.sqrt(s * ((s - side1) * (s - side2) * (s - side3)));
// Log the calculated area to the console
console.log(area);
Output:
14.696938456699069
Live Demo:
See the Pen JavaScript: Area of Triangle - basic-ex-4 by w3resource (@w3resource) on CodePen.
Explanation:
Calculate the area of a triangle of three given sides :
In geometry, Heron's formula named after Hero of Alexandria, gives the area of a triangle by requiring no arbitrary choice of side as base or vertex as origin, contrary to other formulas for the area of a triangle, such as half the base times the height or half the norm of a cross product of two sides.
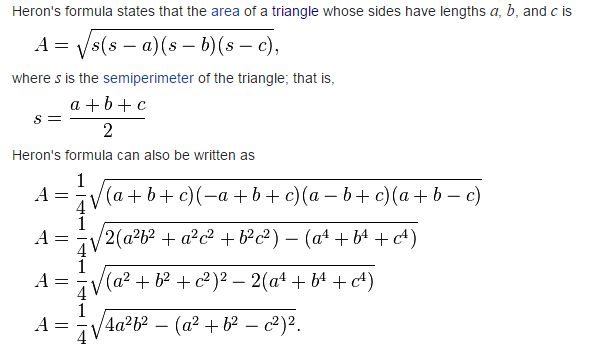
The Math.sqrt() function is used to get the square root of a number. If the value of the number is negative, Math.sqrt() returns NaN.
ES6 Version:
// Declare constants for the lengths of the three sides of a triangle
const side1 = 5;
const side2 = 6;
const side3 = 7;
// Calculate the semi-perimeter of the triangle
const perimeter = (side1 + side2 + side3) / 2;
// Use Heron's formula to calculate the area of the triangle
const area = Math.sqrt(perimeter * ((perimeter - side1) * (perimeter - side2) * (perimeter - side3)));
// Log the calculated area to the console
console.log(area);
For more Practice: Solve these Related Problems:
- Write a JavaScript program to calculate the area of a triangle using Heron’s formula with sides provided by the user.
- Write a JavaScript program that validates the triangle side lengths before calculating the area to ensure they form a valid triangle.
- Write a JavaScript program that computes the area of a triangle given an array of side lengths, checking for the triangle inequality.
Go to:
PREV : Get Current Date in Various Formats.
NEXT : Rotate String 'w3resource' Periodically.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.