JavaScript: Create a new string from a given string, removing the first and last characters of the string if the first or last character are 'P'
JavaScript Basic: Exercise-67 with Solution
Remove First/Last 'P' Characters in String
Write a JavaScript program to create a new string from a given string. This program removes the first and last characters of the string if the first or last character is 'P'. Return the original string if the condition is not satisfied.
The program creates a new string by removing the first and last characters if either is 'P'. If neither condition is met, the program returns the original string.
Visual Presentation:
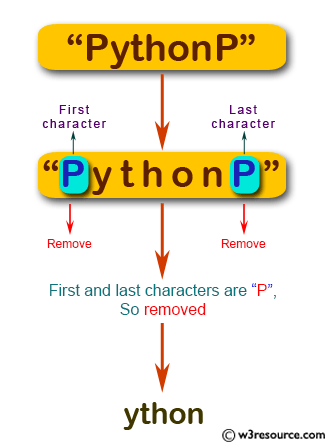
Sample Solution:
JavaScript Code:
// Define a function named nop with parameter str
function nop(str) {
// Initialize start_pos variable to 0
let start_pos = 0;
// Initialize end_pos variable to the length of the input string
let end_pos = str.length;
// Check if the string has a length greater than 0 and the first character is 'P'
if (str.length > 0 && str.charAt(0) == 'P') {
// If true, set start_pos to 1
start_pos = 1;
}
// Check if the string has a length greater than 1 and the last character is 'P'
if (str.length > 1 && str.charAt(str.length - 1) == 'P') {
// If true, decrement end_pos by 1
end_pos--;
}
// Return a substring of the input string from start_pos to end_pos
return str.substring(start_pos, end_pos);
}
// Call the function with sample arguments and log the results to the console
console.log(nop("PythonP"));
console.log(nop("Python"));
console.log(nop("JavaScript"));
Output:
ython ython JavaScript
Live Demo:
See the Pen JavaScript - take the first and last n characters from a given sting - basic-ex-68 by w3resource (@w3resource) on CodePen.
Flowchart:
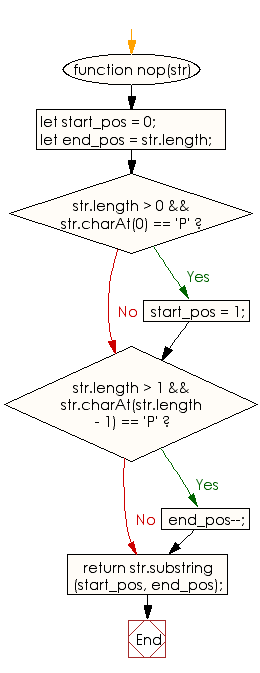
ES6 Version:
// Define a function named nop with parameter str
const nop = (str) => {
// Initialize start_pos and end_pos with default values
let start_pos = 0;
let end_pos = str.length;
// Check if the first character is 'P' and update start_pos if true
if (str.length > 0 && str.charAt(0) === 'P') {
start_pos = 1;
}
// Check if the last character is 'P' and update end_pos if true
if (str.length > 1 && str.charAt(str.length - 1) === 'P') {
end_pos--;
}
// Return the substring based on start_pos and end_pos
return str.substring(start_pos, end_pos);
};
// Call the function with sample arguments and log the results to the console
console.log(nop("PythonP"));
console.log(nop("Python"));
console.log(nop("JavaScript"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that removes the first and last characters of a string if they are the letter 'P' (case-insensitive), and returns the modified string.
- Write a JavaScript function that checks if the first or last character of a string equals 'P' or 'p' and removes them accordingly.
- Write a JavaScript program that processes a string by conditionally stripping off the boundary 'P' characters, leaving the string unchanged if the condition is unmet.
PREV : Return City Name if Starts with 'Los' or 'New'.
NEXT : Use First and Last 'n' Characters from String.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.