JavaScript: Test whether a given array of integers contains 30 or 40 twice
JavaScript Basic: Exercise-79 with Solution
Check if Array Contains 30 and 40 Twice
Write a JavaScript program to test whether a given array of integers contains 30 or 40 twice. The array length should be 0, 1, or 2.
Visual Presentation:
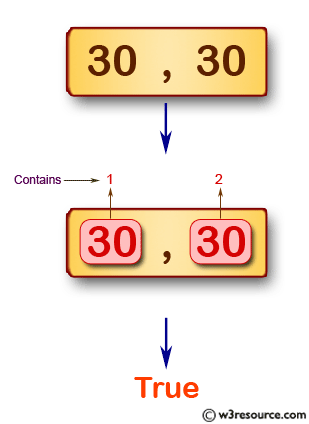
Sample Solution:
JavaScript Code:
// Function to check if the first two elements of an array are either both 30 or both 40
function twice3040(arra1) {
let a = arra1[0], // Extract the first element
b = arra1[1]; // Extract the second element
return (a === 30 && b === 30) || (a === 40 && b === 40); // Check if both are 30 or both are 40
}
// Example usage
console.log(twice3040([30, 30])); // Should return true
console.log(twice3040([40, 40])); // Should return true
console.log(twice3040([20, 20])); // Should return false
console.log(twice3040([30])); // Should return false
Output:
true true false false
Live Demo:
See the Pen JavaScript - Test whether an array of integers of length 2 does not contain 1 or a 3-basic-ex-78 by w3resource (@w3resource) on CodePen.
Flowchart:
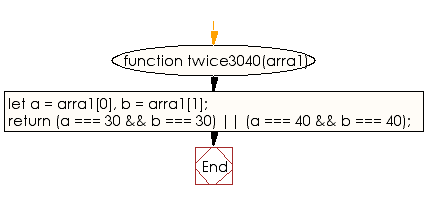
ES6 Version:
// ES6 version using destructuring assignment and arrow function
const twice3040 = ([a, b]) => (a === 30 && b === 30) || (a === 40 && b === 40);
// Example usage
console.log(twice3040([30, 30]));
console.log(twice3040([40, 40]));
console.log(twice3040([20, 20]));
console.log(twice3040([30]));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if an array contains the numbers 30 and 40 exactly twice each, regardless of order.
- Write a JavaScript function that counts occurrences of 30 and 40 in an array and returns true if both appear exactly two times.
- Write a JavaScript program that validates an array by ensuring that the numbers 30 and 40 each occur twice, handling arrays of varying lengths.
- Write a JavaScript function that uses a frequency map to verify that both 30 and 40 have a count of 2 in the array.
Improve this sample solution and post your code through Disqus.
Previous: JavaScript program to test whether an array of integers of length 2 does not contain 1 or a 3.
Next: JavaScript program to swap the first and last elements of a given array of integers. The array length should be at least 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.