JavaScript: Replace each character of a given string by the next one in the English alphabet
JavaScript Basic: Exercise-84 with Solution
Replace Characters with Next in Alphabet
Write a JavaScript program to replace each character in a given string with the next in the English alphabet.
Note: ‘a’ will be replace by ‘b’ or ‘z’ would be replaced by ‘a’.
Visual Presentation:
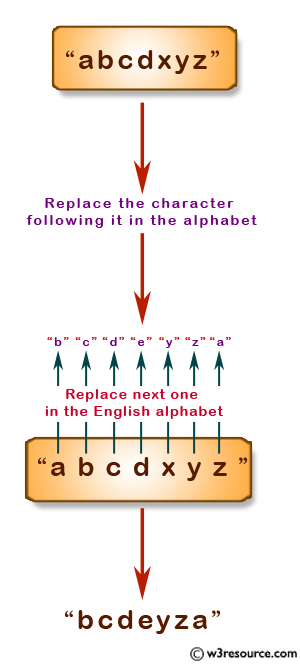
Sample Solution:
JavaScript Code:
// Function to shift each alphabet character in the input string to the next character in the alphabet
function alphabet_char_Shift(str) {
var all_chars = str.split(""); // Convert the input string into an array of characters
for(var i = 0; i < all_chars.length; i++) { // Iterate over each character in the array
var n = all_chars[i].charCodeAt() - 'a'.charCodeAt(); // Calculate the position of the character in the alphabet
n = (n + 1) % 26; // Shift the position to the next character in the alphabet, considering circular nature
all_chars[i] = String.fromCharCode(n + 'a'.charCodeAt()); // Update the character with the shifted character
}
return all_chars.join(""); // Convert the array of characters back to a string and return
}
// Example usage
console.log(alphabet_char_Shift("abcdxyz"));
Output:
bcdeyza
Explanation:
In the exercise above,
- The input string 'str' is split into an array of individual characters using the "split()" method and stored in the variable 'all_chars'.
- A "for" loop iterates over each character in the 'all_chars' array.
- For each character,
- Its Unicode value is obtained using the "charCodeAt()" method, and the Unicode value of 'a' is subtracted to determine its position in the alphabet.
- The position is shifted to the next character in the alphabet using modular arithmetic ((n + 1) % 26). This ensures that if the character is 'z', it wraps around to 'a'.
- The shifted position is converted back to the corresponding character using "String.fromCharCode()" and 'a''s Unicode value is added back.
- The original character in the array is replaced with the shifted character.
- Finally, the "join()" method is used to join the array of characters back into a single string.
Live Demo:
See the Pen javascript-basic-exercise-84 by w3resource (@w3resource) on CodePen.
Flowchart:
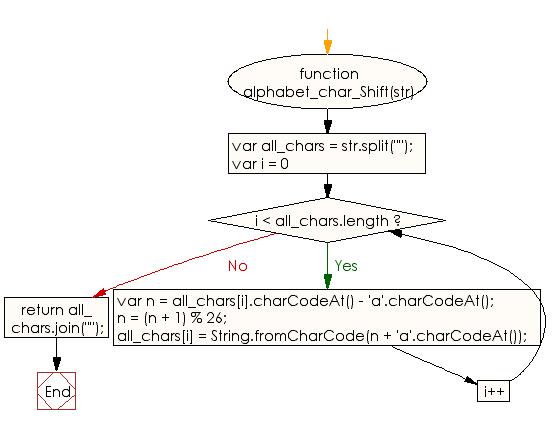
ES6 Version:
// ES6 version with comments
// Function to shift each alphabet character in a string to the next one in the alphabet
const alphabet_char_Shift = (str) => {
var all_chars = str.split(""); // Convert the string to an array of characters
for(var i = 0; i < all_chars.length; i++) {
var n = all_chars[i].charCodeAt() - 'a'.charCodeAt(); // Get the position of the character in the alphabet
n = (n + 1) % 26; // Shift to the next character, considering the cyclic nature of the alphabet
all_chars[i] = String.fromCharCode(n + 'a'.charCodeAt()); // Convert the position back to a character and update the array
}
return all_chars.join(""); // Join the array back to a string and return
};
// Example usage
console.log(alphabet_char_Shift("abcdxyz"));
Improve this sample solution and post your code through Disqus.
Previous: JavaScript to find the longest string from an given array of strings.
Next: JavaScript code to divide an given array of positive integers into two parts.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-basic-exercise-84.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics